【正则表达式简化回文判断】:Java中的高效字符串处理技巧
发布时间: 2024-09-11 01:49:51 阅读量: 61 订阅数: 50 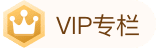
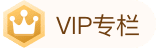
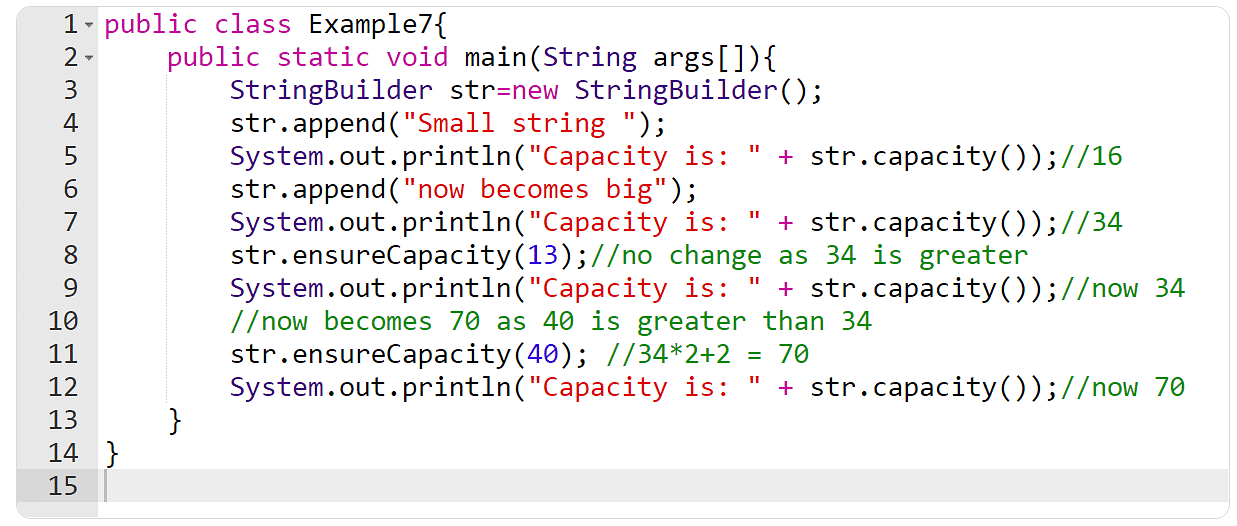
# 1. 正则表达式的基础和应用
## 简介
正则表达式是一种用于匹配字符串中字符组合的模式。在文本处理中,它们是强大的工具,能够通过一组简单的语法规则,帮助我们快速找到符合特定规则的字符串片段。
## 基础语法
正则表达式的基本元素包括普通字符、特殊字符、元字符等。普通字符直接代表自己,特殊字符如点号(`.`)和星号(`*`)表示匹配任意字符和重复前一个字符,而元字符如问号(`?`)和加号(`+`)用于定义重复的模式。
## 应用场景
在编程和文本编辑中,正则表达式被广泛用于数据验证、文本提取和替换等场景。例如,通过正则表达式,我们可以快速验证电子邮件地址的格式,或是从大量的日志文件中提取错误信息。
```java
import java.util.regex.Pattern;
public class RegexExample {
public static void main(String[] args) {
Pattern pattern = ***pile("^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$");
boolean matches = pattern.matcher("***").matches();
System.out.println(matches); // 输出: true
}
}
```
以上示例代码使用了Java的`Pattern`类来编译一个正则表达式,并用其`matcher`方法检查一个字符串是否符合电子邮件格式的规则。输出结果为`true`,表示这个字符串是一个有效的电子邮件地址。
# 2. 字符串处理技巧与正则表达式
### 2.1 字符串处理的基本概念
#### 2.1.1 字符串的定义与重要性
字符串是编程中常见的一种数据类型,它是由字符组成的序列,用于表示文本信息。字符串的重要性不言而喻,从简单的用户输入验证到复杂的文本分析处理,字符串的处理无处不在。在IT行业中,无论是数据处理、日志分析还是用户交互,良好的字符串处理技巧都是必不可少的。
#### 2.1.2 Java中的字符串处理方法
Java为字符串提供了丰富的处理方法,例如`concat()`, `substring()`, `replace()`, `trim()`等。除此之外,Java也提供了一系列的`StringBuilder`和`StringBuffer`类,用于高效地构建和修改字符串。要熟练掌握这些方法,有助于我们编写出更高效、更简洁的代码。
```java
// 示例:Java中的字符串处理
String originalString = "Hello, World!";
String upperCaseString = originalString.toUpperCase(); // 转换为大写
String trimmedString = originalString.trim(); // 去除前后空白
String subString = originalString.substring(7, 12); // 截取子字符串
System.out.println("Upper Case: " + upperCaseString);
System.out.println("Trimmed: " + trimmedString);
System.out.println("Substring: " + subString);
```
上述代码展示了如何在Java中使用基本的字符串处理方法。
### 2.2 正则表达式在字符串处理中的作用
#### 2.2.1 正则表达式的定义和组成
正则表达式(Regular Expression,简称Regex)是一种文本模式,包括普通字符(例如,字母和数字)和特殊字符(称为"元字符")。它提供了一种灵活且强大的方式来搜索或替换符合特定模式的文本。
#### 2.2.2 正则表达式在Java中的应用实例
在Java中,我们可以利用`java.util.regex`包中的`Pattern`和`Matcher`类来处理正则表达式。下面的实例展示了如何在Java中使用正则表达式来匹配一个电子邮件地址格式。
```java
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class RegexExample {
public static void main(String[] args) {
String regex = "^[\\w-\\.]+@([\\w-]+\\.)+[\\w-]{2,4}$";
String stringToTest = "***";
Pattern pattern = ***pile(regex);
Matcher matcher = pattern.matcher(stringToTest);
if (matcher.matches()) {
System.out.println("Email address is valid");
} else {
System.out.println("Email address is not valid");
}
}
}
```
这个简单的程序展示了如何使用正则表达式来验证电子邮件地址的格式。正则表达式`^[\\w-\\.]+@([\\w-]+\\.)+[\\w-]{2,4}$`用于匹配典型的电子邮件地址格式。
### 2.3 理解正则表达式的元字符和构造
#### 2.3.1 常用元字符的介绍
元字符在正则表达式中具有特殊的意义。例如,`.`表示任意单个字符,`*`表示前面的元素可以出现零次或多次,`+`表示一次或多次,而`?`表示零次或一次。这些元字符为构建复杂的模式提供了基础。
#### 2.3.2 字符类、量词和锚点的使用
字符类允许我们定义一组字符,其中任何一个字符都可以匹配,例如`[a-zA-Z]`匹配任何小写或大写英文字母。量词如`{n,m}`表示前面的元素至少出现n次,最多出现m次。锚点`^`和`$`用于匹配字符串的开始和结束,确保模式匹配整个字符串而不是字符串的一部分。
```java
// 示例:使用元字符和构造
String text = "The quick brown fox jumps over the lazy dog";
String regex = "^[Tt]he.*[dD]og$";
Pattern pattern = ***pile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.matches()) {
System.out.println("Text matches the regex");
} else {
System.out.println("Text does not match the regex");
}
```
上述代码中的正则表达式`^[Tt]he.*[dD]og$`用于匹配以"The"或"the"开头,并以"dog"或"Dog"结尾的字符串。
### 2.4 实际应用中的正则表达式技巧
正则表达式不仅仅是理论上的概念,它们在实际应用中也非常重要。例如,数据清洗、日志分析和网络爬虫都会频繁使用到正则表达式。掌握正则表达式的高级技巧,如前瞻断言、后顾断言和正向负向零宽断言等,能够解决更多复杂的问题。
```java
// 示例:使用断言
String content = "Example: is it working?";
// 前瞻断言,查找后面跟着冒号和空格的单词
String regexWithLookahead = "\\b(\\w+)(?=\\s:\\s)";
Pattern patternWithLookahead = ***pile(regexWithLookahead);
Matcher matcherWithLookahead = patternWithLookahead.matcher(content);
while (matcherWithLookahead.find()) {
System.out.println("Found: " + matcherWithLookahead.group(1));
}
// 后顾断言,查找前面是问号的单词
String regexWithLookbehind = "(?<=\\?)\\b(\\w+)";
Pattern patternWithLookbehind = ***pile(regexWithLookbehind);
Matcher matcherWithLookbehind = patternWithLookbehind.matcher(content);
while (matcherWithLookbehind.find()) {
System.out.println("Found: " + matcherWithLookbehind.group(1));
}
```
上述代码展示了如何使用Java中的`Pattern`和`Matcher`类结合前瞻和后顾断言来提取特定模式的文本。
通过这些实例,我们可以看出正则表达式在字符串处理中
0
0
相关推荐
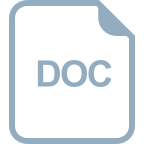
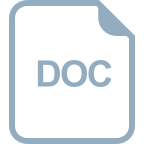
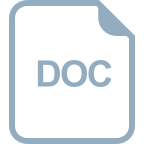
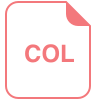
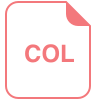
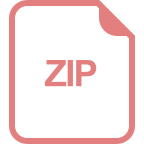
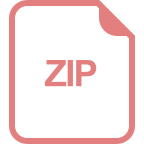
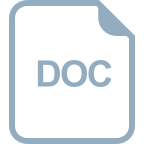
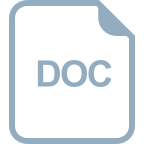