【Java程序员面试指南:回文问题准备秘籍】
发布时间: 2024-09-11 01:24:44 阅读量: 30 订阅数: 50 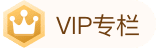
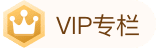
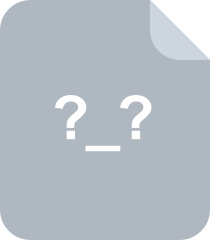
1155:回文三位数.cpp
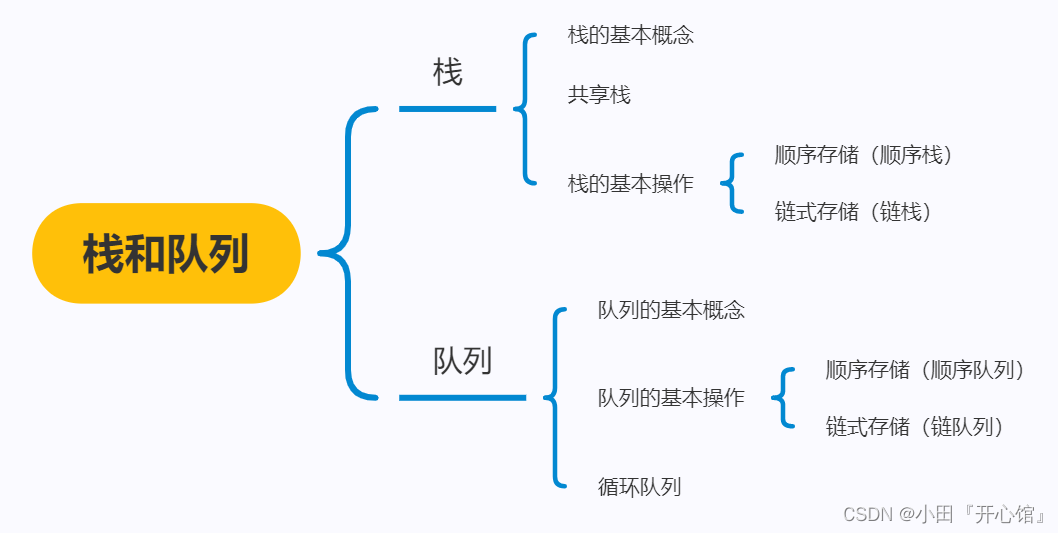
# 1. 回文问题概述
回文,顾名思义,是指一个字符串正读和反读都相同的序列。从古老的对联到现代的数字世界,回文都扮演着一个有趣而重要的角色。本章将为读者介绍回文的基本概念、它的历史渊源以及在现代科技领域中的应用。
## 1.1 回文的定义
回文是一种特殊的字符串序列,正着读和反着读是完全相同的。例如,"madam"或"racecar"就是回文的例子。回文的概念不仅仅局限于英文字母,它也适用于数字和其它语言字符。
## 1.2 回文的识别
识别回文的最直观方法就是将字符串进行反转,然后和原字符串进行比较。如果两者完全相同,那么这个字符串就是一个回文。在编程实现中,这样的方法虽然简单,但在处理较长的字符串时,效率会比较低。
## 1.3 回文在科技中的应用
在计算机科学和信息处理领域,回文的特性被广泛应用于各种算法中,例如文本编辑、数据库索引、生物信息学等。它们不仅在理论研究中有重要地位,而且在实际应用中也体现出了极大的价值。
通过本章的概述,读者将对回文问题有一个初步的认识,为后续章节深入探讨回文问题的理论基础和编程实现打下坚实的基础。
# 2. 回文问题的理论基础
### 2.1 回文的定义和分类
#### 2.1.1 简单回文的概念
简单回文是指一个字符串从前往后读和从后往前读是完全相同的,例如“radar”、“level”或“deified”。这种回文结构简单,但却是许多复杂回文的基础。在编程实现时,我们通常需要关注字符串的首尾字符是否相同,以及中间是否存在对称性。
#### 2.1.2 复杂回文的特点
复杂回文不仅包括简单回文,还包括那些需要通过一些变换才能发现对称性的字符串。例如,通过忽略空格和标点符号,单词序列“a man, a plan, a canal, Panama”也能被视为回文。处理复杂回文问题时,程序需要能够跳过非字母字符,并且正确处理大小写变化。
### 2.2 回文检测的算法原理
#### 2.2.1 字符串遍历方法
最直接的回文检测方法是通过从字符串两端向中心遍历,比较对应的字符是否相同。对于简单回文,该方法的效率相对较高,时间复杂度为O(n/2),即O(n)。以下是使用Java实现的一个示例代码块:
```java
public boolean isPalindromeSimple(String s) {
int left = 0; // 字符串左端的索引
int right = s.length() - 1; // 字符串右端的索引
while (left < right) {
if (s.charAt(left) != s.charAt(right)) {
return false; // 如果字符不相同,则不是回文
}
left++; // 向中心移动
right--; // 向中心移动
}
return true; // 所有字符都相同,是回文
}
```
#### 2.2.2 栈和队列在回文检测中的应用
为了处理更复杂的回文问题,我们可以使用栈或队列来保存中间结果。栈是后进先出(LIFO)的数据结构,可以用来存储字符串的一半字符,然后再从栈中弹出字符与另一半进行比较。这种方法可以有效处理字符串长度为奇数和偶数的情况。以下是利用栈进行回文检测的示例代码:
```java
public boolean isPalindromeStack(String s) {
Stack<Character> stack = new Stack<>();
int left = 0;
int right = s.length() - 1;
while (left < right) {
stack.push(s.charAt(left++)); // 将左半部分字符压入栈
stack.push(s.charAt(right--)); // 将右半部分字符压入栈
}
while (!stack.isEmpty() && left <= right) {
char stackTop = stack.pop(); // 从栈顶弹出字符
if (stackTop != s.charAt(left++)) {
return false; // 如果字符不匹配,则不是回文
}
}
return stack.isEmpty(); // 如果栈为空,则是回文
}
```
#### 2.2.3 时间复杂度分析
在分析回文检测算法的时间复杂度时,需要注意算法中循环的次数和每次循环的操作。例如,字符串遍历方法的时间复杂度是O(n),因为它需要遍历整个字符串一次。而栈或队列的方法,理论上时间复杂度也是O(n),但其空间复杂度会因为需要额外的空间来保存字符而增加。
### 2.3 回文问题的数学模型
#### 2.3.1 中心扩展法和动态规划
为了优化回文检测的效率,我们可以使用中心扩展法。这种方法的核心思想是从字符串中心开始向外扩展,检查每个可能的回文中心。动态规划则是另一种优化方法,通过构建一个二维表来记录字符串的子串是否为回文,从而避免重复计算。
#### 2.3.2 数学证明与理论极限
回文问题的数学模型有助于我们理解问题的本质,并找到解决问题的更优策略。证明回文的存在性、唯一性或构造性,以及计算回文检测的时间复杂度上限,都是理论研究的重要部分。然而,由于回文问题在最坏情况下的时间复杂度为O(n^2),因此寻找更快的算法是研究者们面临的一个挑战。
以上是对第二章回文问题的理论基础部分的详细介绍。接下来,我们将探讨回文问题的编程实现,包括基础编程语言的选择、环境配置、回文检测算法的实现以及代码的优化和性能测试。
# 3. 回文问题的编程实现
## 3.1 基础编程语言选择与环境配置
### 3.1.1 Java环境搭建
在开始回文问题的编程实现之前,我们需要选择合适的编程语言和环境配置。考虑到跨平台和强大的社区支持,Java是一个不错的选择。下面是Java环境搭建的详细步骤:
1. **下载JDK**:首先需要访问Oracle官网或OpenJDK下载最新的JDK版本。
2. **安装JDK**:根据操作系统不同,执行相应的安装程序。
3. **配置环境变量**:
- `JAVA_HOME`:指向JDK的安装目录。
- `PATH`:添加`%JAVA_HOME%\bin`(Windows)或`$JAVA_HOME/bin`(Mac/Linux)。
验证安装是否成功,在命令行输入`java -version`和`javac -version`,应该能看到JDK的版本信息。
### 3.1.2 开发工具的选择
选择一个合适的集成开发环境(IDE)可以提高开发效率。常见的Java IDE有:
- **IntelliJ IDEA**:功能全面,社区版免费。
- **Eclipse**:插件丰富,开源且免费。
- **NetBeans**:易于使用,同样开源免费。
这里以IntelliJ IDEA为例进行开发环境配置:
1. 下载并安装IntelliJ IDE
0
0
相关推荐
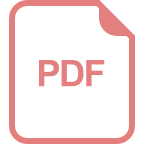
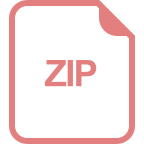
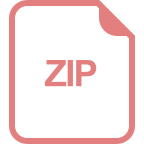
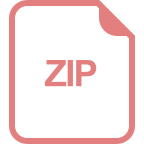
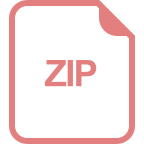
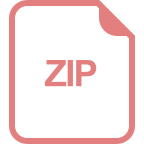
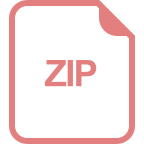
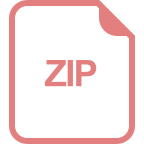