【动态规划:Java实现回文检测的高级解决方案】
发布时间: 2024-09-11 01:34:24 阅读量: 41 订阅数: 50 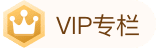
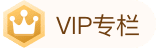
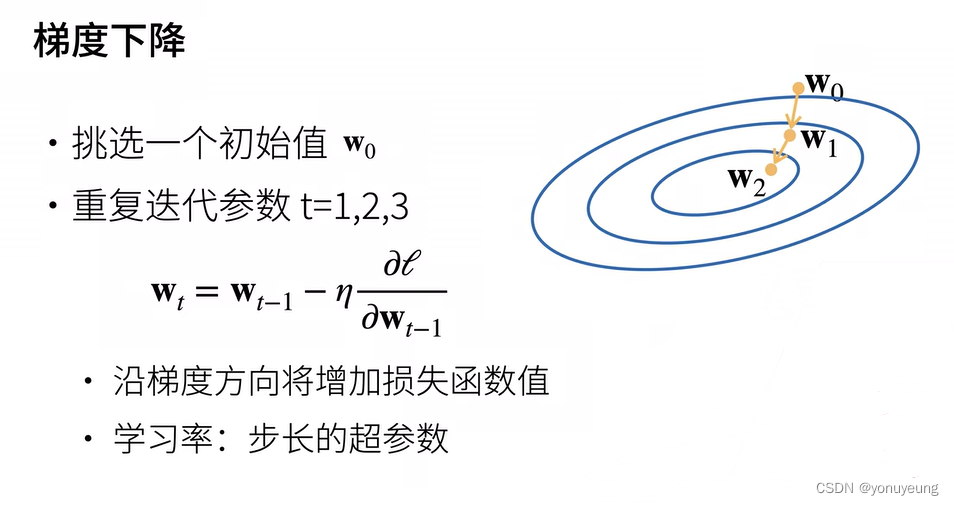
# 1. 动态规划原理与回文基础
## 1.1 动态规划概述
动态规划是一种算法设计技术,它将一个问题分解成相互关联的子问题,并存储这些子问题的解(通常是在一个表格中),以避免重复计算。这种方法特别适用于具有重叠子问题和最优子结构特性的问题。
## 1.2 回文的定义
回文是指正读和反读都相同的字符串,比如“radar”或“level”。在动态规划中,回文检测是理解和实现动态规划的一个绝佳起点,因为其子问题关系和解决方案的递归性质。
## 1.3 动态规划解法优势
动态规划方法可以有效避免重复计算子问题,提高效率。特别是在检测大型字符串是否为回文时,这种方法比简单的字符串反转比较具有明显的时间优势。
## 1.4 动态规划在回文检测中的应用
例如,动态规划可以用来检测字符串是否为回文。通过比较字符串两端的字符是否相同,然后在字符串中移除两端相同的字符继续这个过程,我们可以将问题分解成更小的子问题,并存储中间结果来提升算法效率。
```java
boolean isPalindrome(String str) {
// dp[i][j] 表示从索引i到j的子字符串是否为回文
boolean[][] dp = new boolean[str.length()][str.length()];
for (int i = str.length() - 1; i >= 0; i--) {
for (int j = i; j < str.length(); j++) {
if (str.charAt(i) == str.charAt(j)) {
if (j - i < 2 || dp[i+1][j-1]) {
dp[i][j] = true;
}
}
}
}
return dp[0][str.length()-1];
}
```
动态规划是理解和实现高效回文检测的关键,为后续章节中更高级的应用和优化打下了基础。
# 2. Java中的动态规划算法实现
在讨论动态规划之前,我们先来简要回顾一下动态规划的原理,并探索回文检测问题的动态规划解法。
## 2.1 动态规划与回文检测
### 2.1.1 动态规划的基本概念
动态规划(Dynamic Programming,DP)是一种算法设计技巧,用于求解具有重叠子问题和最优子结构特性的问题。在动态规划中,问题被分解为较小子问题,并将子问题的解保存下来,避免重复计算,最终合并子问题的解得到原问题的解。
动态规划解决问题的基本步骤通常包括:
1. 定义子问题。
2. 实现递归关系。
3. 计算子问题的解并保存它们,通常保存在一个表格中,这个表格被称为动态规划表格。
4. 根据表格中的解构建最终解。
### 2.1.2 回文检测问题的动态规划解法
回文检测问题的目标是判断一个字符串是否是回文字符串。回文字符串是正读和反读都相同的字符串。我们可以通过动态规划来解决这个问题。
假设 `s` 是一个字符串,那么动态规划的状态 `dp[i][j]` 可以表示字符串从索引 `i` 到 `j` 是否构成回文。状态转移方程如下:
```
dp[i][j] = (s[i] == s[j]) && (j - i < 3 || dp[i+1][j-1])
```
这个方程说明,只有当两个字符相同,并且字符串长度小于等于 3 或者内部的子字符串是回文时,整体字符串才是回文。
## 2.2 代码实现与优化
### 2.2.1 构建动态规划表格
接下来,我们将根据上述思路用Java代码实现动态规划解法:
```java
public static boolean isPalindrome(String s) {
int n = s.length();
boolean[][] dp = new boolean[n][n];
for (int i = n - 1; i >= 0; i--) {
for (int j = i; j < n; j++) {
dp[i][j] = (s.charAt(i) == s.charAt(j)) && (j - i < 3 || dp[i + 1][j - 1]);
}
}
return dp[0][n - 1];
}
```
在这段代码中,我们首先初始化了一个二维数组 `dp`,然后从字符串的末尾开始,逐步填充 `dp` 表格。我们注意当 `j - i < 3` 时,如果两端字符相等,那么中间最多只有一个字符,所以可以确定是回文。
### 2.2.2 优化空间复杂度
在上述实现中,空间复杂度为 O(n^2),我们可以通过分析得知,每次状态转移仅依赖于 `dp[i+1][j-1]`,所以我们可以使用一维数组来记录状态,实现空间复杂度的优化。
### 2.2.3 提升算法效率
在代码实现中,我们通过自底向上的方式填充动态规划表格,每完成一个 `dp[i][j]` 的计算,就能够立即用于计算上层的 `dp` 状态。这样可以避免递归实现中可能出现的重复计算。
整个算法的时间复杂度是 O(n^2),这是因为我们需要考虑字符串中每一对可能的字符位置组合。
### 表格展示
我们用一个表格来展示 `dp` 数组的变化过程。这里,表格的行和列分别代表字符串的不同位置,值为 `true` 或者 `false`,表示对应位置是否构成回文。
| | 0 | 1 | 2 | 3 | 4 | 5 | 6 |
|-----|-----|-----|-----|-----|-----|-----|-----|
| 0 | true| - | - | - | - | - | true|
| 1 | - | true| - | - | - | true| - |
| 2 | - | - | true| - | true| - | - |
| 3 | - | - | - | true| - | - | - |
| 4 | - | - | true| - | true| - | - |
| 5 | - | true| - | - | - | true| - |
| 6 | true| - | - | - | - | - | true|
(注:表格中的 `true` 和 `false` 表示两个索引之间的子字符串是否是回文)
### Mermaid 流程图
下面是用Mermaid语法描述的动态规划解决问题流程图:
```mermaid
graph TD
A[开始] --> B[初始化DP数组]
B --> C[字符串长度n]
C --> D{字符串长度小于等于1}
D -- 是 --> E[直接返回true]
D -- 否 --> F[逐个填充DP数组]
F --> G[子问题DP[i+1][j-1]]
G --> H{字符串i到j是否相等}
H -- 是 --> I[更新DP[i][j]]
I --> F
H -- 否 --> F
G --> J[返回DP[0][n-1]状态]
```
(注:上述流程图表示了动态规划算法从初始化DP数组到返回最终结果的处理步骤)
通过这些步骤,我们实现了一个基于动态规划的回文检测算法,它具有线性时间复杂度的特性,并且能够高效地处理大量字符串数据。动态规划不仅是一种编程技巧,它在算法设计中具有非常重要的作用,帮助我们理解和优化复杂问题的解决方案。
# 3. 回文检测的Java实践
## 3.1 字符串处理技巧
### 3.1.1 字符串遍历与索引管理
在Java中,处理字符串通常涉及到遍历字符以及索引的精确管理。字符串遍历可以通过`for`循环、`for-each`循环或者使用迭代器等方式完成。字符的遍历通常是基于字符数组或者直接利用`String`类提供的方法。
```java
String str = "abccba";
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
// 处理每个字符
}
```
在上面的代码块中,`for`循环用于遍历字符串中的每个字符。利用`charAt(i)`方法获取位置`i`处的字符。索引`i`从`0`开始,直到`str.length() - 1`,保证不越界。
索引管理是回文检测中非常关键的部分。正确的索引管理能够保证回文检测算法正确地比较字符串的对称位置。对于长度为`n`的字符串,回文检测的索引范围是`0`到`n/2`。
### 3.1.2 字符串反转与比较
字符串反转是回文检测的一个常见步骤。在Java中,可以通过简单的循环实现字符串的反转。
```java
StringBuilder sb = new StringBuilder(str);
String reversedStr = sb.reverse().toString();
```
字符串反转后,比较原字符串与反转后的字符串是判断回文的直接方法。若两者相等,则字符串是回文;否则,不是。
```java
boolean isPalindrome = str.e
```
0
0
相关推荐
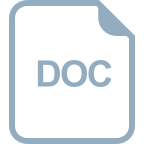
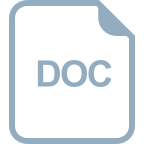
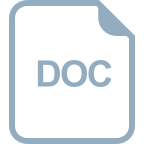
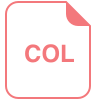
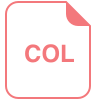
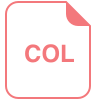
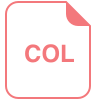
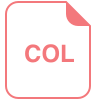
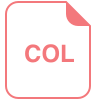