【从回文判断到构造:Java技术进阶】:掌握回文字符串的生成艺术
发布时间: 2024-09-11 01:10:39 阅读量: 67 订阅数: 50 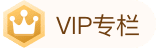
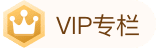
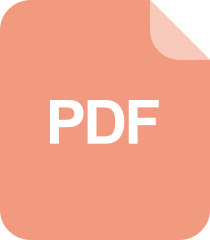
Java判断字符串回文的代码实例


# 1. 回文字符串基础介绍
回文字符串是字符串领域中的一个基础且非常有趣的概念。它指的是一个字符串正读和反读都相同的特性,例如 "radar" 或 "level"。回文不仅在文学作品中被广泛用以构建对称的美学效果,而且在计算机科学领域,特别是在算法设计和数据结构优化中也有重要的应用。
理解回文字符串对于初学者而言是一个很好的起点,因为它可以帮助我们建立算法的基本功。回文的判断与构造,贯穿了算法设计的诸多基本原理,如动态规划、贪心算法和字符串处理等。掌握了这些概念,我们才能更好地应对更高级的编程挑战,如字符串匹配、编辑距离等复杂问题。
在接下来的章节中,我们将深入探讨回文字符串相关的算法原理与实现策略,以及如何在实际应用中发挥其独特的价值。通过学习这些内容,读者将能够提升编程能力,并在软件开发中实现更加高效和优雅的解决方案。
# 2. ```
# 第二章:回文判断的算法原理与实现
## 2.1 回文的定义与性质
### 2.1.1 回文的数学定义
回文是指一个字符串正读和反读都相同的字符串。例如,"madam"或"racecar"都是回文。从数学的角度来看,一个字符串是回文的,当且仅当它在某个特定的点被分割成两个部分时,前半部分与后半部分是镜像对称的。
为了更精确地描述,设字符串S的长度为n,如果对于所有的i(0 <= i < n/2),有S[i] = S[n-i-1],那么字符串S是回文的。这个定义为我们实现回文判断提供了数学基础。
### 2.1.2 回文在计算机科学中的应用
在计算机科学中,回文的概念不仅限于字符串处理。例如,在文本编辑器中,实现回文匹配可以帮助快速定位到文本中的特定模式。在数据结构中,回文树和回文链表等是特殊类型的结构,它们在某些算法中有着重要的应用。
回文的一个重要应用是数据加密。传统的回文加密算法将数据和它的逆序进行某种形式的结合,这可以用于生成某些特定类型的伪随机数序列。除此之外,回文还常用于设计特定的哈希函数和校验码。
## 2.2 字符串反转算法
### 2.2.1 简单字符串反转的实现
最直观的字符串反转方法是创建一个新字符串,并从原字符串的末尾开始逐个字符地将字符添加到新字符串的末尾。以下是使用Java实现的简单字符串反转示例代码:
```java
public static String reverseString(String input) {
StringBuilder sb = new StringBuilder(input);
return sb.reverse().toString();
}
```
这段代码首先通过`StringBuilder`将原始字符串转换成一个可变的字符序列,接着使用`reverse`方法将字符序列反转,最后再将反转后的字符序列转换回字符串。
### 2.2.2 利用栈实现字符串反转
使用栈数据结构可以以不同的方式实现字符串反转。栈的后进先出(LIFO)特性很适合处理这种类型的反转问题。Java中可以通过`Stack`类实现,或者更现代的方法是使用`Deque`接口。
```java
public static String reverseStringWithStack(String input) {
Deque<Character> stack = new ArrayDeque<>();
for (char c : input.toCharArray()) {
stack.push(c);
}
StringBuilder sb = new StringBuilder();
while (!stack.isEmpty()) {
sb.append(stack.pop());
}
return sb.toString();
}
```
这段代码首先遍历输入字符串,将每个字符推入栈中。然后,通过弹出栈顶元素的方式将字符逐个添加到`StringBuilder`中,这样就实现了字符的反转。
## 2.3 回文判断的实现策略
### 2.3.1 双指针技术
双指针技术是处理字符串和数组中对称问题的一种常用方法。在判断回文时,我们可以使用一个指针从字符串的开始位置出发,另一个指针从字符串的末尾开始出发,然后逐个字符比较这两个指针所指向的字符。
如果所有对称位置的字符都相等,那么这个字符串就是回文。以下是使用Java实现的回文判断代码:
```java
public static boolean isPalindrome(String s) {
int left = 0;
int right = s.length() - 1;
while (left < right) {
if (s.charAt(left) != s.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
```
这段代码首先初始化两个指针,分别指向字符串的起始位置和结束位置。然后,通过一个循环逐个比较两个指针所指字符是否相等,如果不相等则立即返回`false`。如果所有字符都相等,循环结束后返回`true`。
### 2.3.2 动态规划方法
动态规划是一种通过把原问题分解为相对简单的子问题的方式求解复杂问题的方法。在回文判断中,可以使用动态规划来避免重复计算之前已经确定为回文的子串。
为了使用动态规划,我们可以创建一个二维数组`dp`,其中`dp[i][j]`表示从索引`i`到`j`的子串是否是回文。首先初始化所有长度为1的子串都是回文,然后通过逐渐增加子串长度的方式,填充`dp`数组。
```java
public static boolean isPalindromeWithDP(String s) {
int n = s.length();
boolean[][] dp = new boolean[n][n];
for (int i = 0; i < n; i++) {
dp[i][i] = true;
}
for (int len = 2; len <= n; len++) {
for (int start = 0; start <= n - len; start++) {
int end = start + len - 1;
if (s.charAt(start) == s.charAt(end)) {
if (len == 2 || dp[start + 1][end - 1]) {
dp[start][end] = true;
}
}
}
}
return dp[0][n - 1];
}
```
在这段代码中,首先初始化对角线上的元素为`true`,因为单个字符总是回文。然后,我们用两层循环来确定长度从2到n的所有子串。对于每一个子串,如果它两端的字符相同,并且剩余的子串长度为1或者剩余子串也是回文,那么这个子串就是回文。最终,`dp[0][n - 1]`的值就决定了整个字符串是否是回文。
```
# 3. 回文构造的技巧与策略
## 3.1 回文构造的基本概念
### 3.1.1 回文构造问题的定义
回文构造问题通常指的是给定一个字符串,找出其回文子序列的个数,或者构造出一个或多个最长的回文子序列。回文子序列是指在原字符串中按顺序出现,但不需要连续的一组字符组成的序列,可以不必在原字符串中连续,但需要保持字符的相对顺序。
回文构造问题在生物信息学、数据压缩、和自然语言处理等领域都有广泛的应用。比如,在生物信息学中,寻找DNA序列中的回文结构有助于分析和理解基因序列。在数据压缩中,通过回文构造可以发
0
0
相关推荐
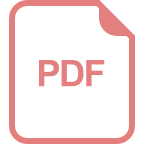
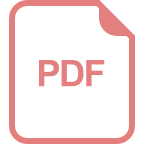
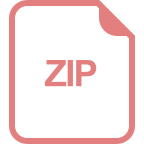
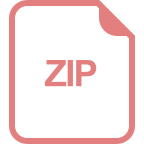
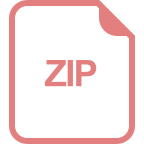
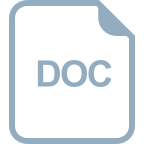
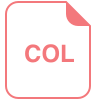

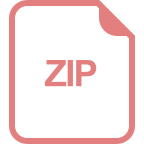