【双指针技术的Java实现】:回文检测的极致简化
发布时间: 2024-09-11 01:07:00 阅读量: 33 订阅数: 23 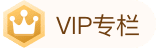
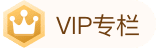
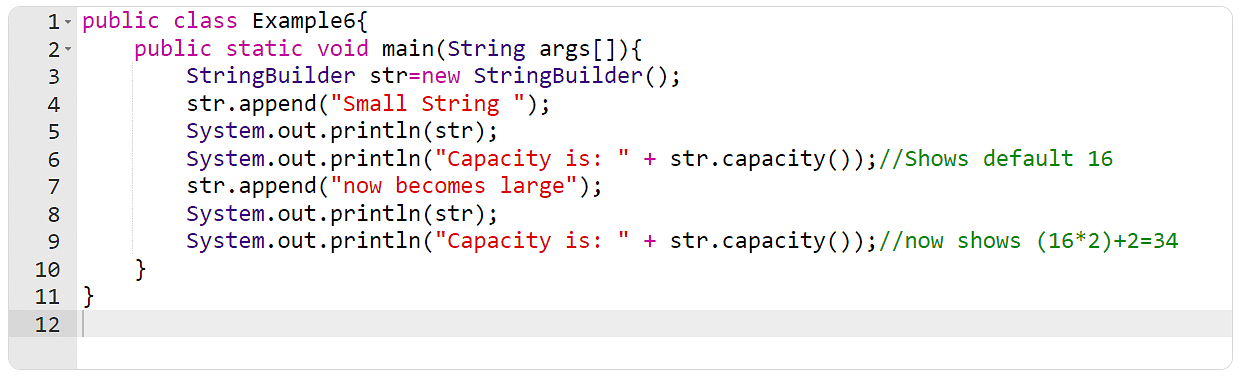
# 1. 双指针技术的基本概念与原理
双指针技术是一种高效的数据处理方法,在数组、链表等线性数据结构操作中应用广泛。该技术主要通过两个指针从不同位置同时遍历数据结构,以实现特定的算法目标,如检测回文、排序、查找等。相较于传统的单指针遍历,双指针技术能够更有效地减少不必要的遍历次数,从而提高算法的时间效率。
在双指针技术中,两个指针可以是"快慢指针",也可以是"前后指针"。快慢指针用于处理如链表中环的检测、排序算法中的稳定快速排序等复杂问题;而前后指针则常用于数组和字符串的查找、修改等操作,如两数之和、最长回文子串等经典问题。
理解双指针技术的原理需要我们掌握指针的概念以及它与数组或链表节点的对应关系。本章将详细介绍双指针技术的基本概念、原理和在数据结构中的应用,为进一步探索双指针技术打下坚实的基础。
# 2. 双指针技术在Java中的应用
## 2.1 双指针技术与Java语言特性
### 2.1.1 Java语言简介
Java是一种广泛使用的面向对象的高级编程语言,自1995年问世以来,它凭借其跨平台性、丰富的类库和成熟的生态系统,成为全球最受欢迎的编程语言之一。Java的设计哲学是“一次编写,到处运行”,这得益于其独特的虚拟机(JVM)架构。JVM能够将Java字节码转换成不同操作系统上的机器码,从而保证了Java程序能够在任何安装了相应JVM的设备上运行。
### 2.1.2 Java中的双指针技术概述
在Java中,双指针技术通常是通过两个引用变量(指针)来实现的,这两个指针可以指向同一个数组或集合中的不同位置。通过合理地移动这两个指针,可以在不增加额外空间复杂度的情况下,高效地解决一系列与数组或集合相关的问题。双指针技术在Java中的应用,充分利用了Java语言提供的引用传递和自动内存管理的特点,能够简化代码逻辑,提高程序性能。
## 2.2 双指针在数组和字符串处理中的应用
### 2.2.1 数组操作中的双指针技巧
在数组操作中,双指针技术经常被用来进行快速排序、合并两个已排序数组以及解决各种滑动窗口问题。例如,在快速排序中,可以使用一个左指针和一个右指针从数组两端向中心扫描,交换不对序的元素,直到两个指针相遇。这种技巧极大地提高了排序的效率。
```java
// 快速排序中交换元素的示例
public static void quickSort(int[] arr, int left, int right) {
if (left >= right) {
return;
}
int i = left, j = right;
while (i < j) {
while (i < j && arr[j] >= arr[left]) j--;
while (i < j && arr[i] <= arr[left]) i++;
if (i < j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i];
arr[i] = arr[left];
arr[left] = temp;
quickSort(arr, left, i - 1);
quickSort(arr, i + 1, right);
}
```
### 2.2.2 字符串处理的双指针策略
在字符串处理中,双指针同样具有重要的作用。在进行字符串匹配、反转、验证回文等问题时,使用双指针策略可以帮助我们用更少的计算量和时间来完成任务。例如,验证一个字符串是否为回文,可以通过两个指针从字符串的两端开始向中间移动,比较对应的字符是否相同,如果在遍历过程中所有的对应字符都相等,则字符串为回文。
```java
// 验证字符串是否为回文的示例
public static boolean isPalindrome(String s) {
int left = 0, right = s.length() - 1;
while (left < right) {
while (left < right && !Character.isLetterOrDigit(s.charAt(left))) {
left++;
}
while (left < right && !Character.isLetterOrDigit(s.charAt(right))) {
right--;
}
if (Character.toLowerCase(s.charAt(left)) != Character.toLowerCase(s.charAt(right))) {
return false;
}
left++;
right--;
}
return true;
}
```
## 2.3 双指针与Java集合框架的结合
### 2.3.1 集合框架中的双指针用法
Java集合框架提供了丰富的方法来操作集合中的元素。然而,在一些复杂问题的处理中,如在集合中查找满足特定条件的元素,双指针技术可以发挥出色的作用。通过双指针,我们可以高效地遍历集合中的元素,例如,使用迭代器快速地定位到满足条件的元素。
```java
import java.util.List;
import java.util.ArrayList;
public static List<Integer> findDuplicatedNumbers(List<Integer> nums) {
List<Integer> duplicates = new ArrayList<>();
if (nums == null || nums.size() <= 1) return duplicates;
int left = 0, right = 1;
while (right < nums.size()) {
if (nums.get(left).equals(nums.get(right))) {
duplicates.add(nums.get(left));
right++;
} else if (left < right) {
left++;
right = left;
} else {
right++;
}
}
return duplicates;
}
```
### 2.3.2 双指针在迭代器中的实现
Java迭代器是集合框架的核心,它允许我们以统一的方式遍历不同类型的集合。在迭代器中使用双指针技巧,可以有效地解决一些特定问题,例如在已排序的集合中快速找到两个元素的和等于指定值的问题。
```java
import java.util.*;
public static List<List<Integer>> findTwoSumPairs(Iterator<Integer> iterator, int target) {
List<List<Integer>> result = new ArrayList<>();
if (iterator == null) return result;
int left = 0, right = 1;
if (iterator.hasNext()) iterator.next();
if (iterator.hasNext()) iterator.next();
while (iterator.hasNe
```
0
0
相关推荐






