使用python编写程序,从新浪财经取得SZ300666的每日分时数据,获取每日最高价和最低价的时间,并且统计60%的最高价时间区间和最低价的时间区间,将该区间打印出来
时间: 2023-12-21 14:05:05 浏览: 27
可以使用Python中的requests和BeautifulSoup库来爬取网页数据。具体的步骤如下:
1. 导入需要的库
```python
import requests
from bs4 import BeautifulSoup
```
2. 构造请求URL,发送GET请求,获取HTML页面
```python
url = 'http://finance.sina.com.cn/realstock/company/sh300666/nc.shtml'
response = requests.get(url)
html = response.content
```
3. 解析HTML页面,获取每日的分时数据
```python
soup = BeautifulSoup(html, 'html.parser')
data_table = soup.find_all('table', class_='datatbl')[0]
data_rows = data_table.find_all('tr')[1:]
data = []
for row in data_rows:
cols = row.find_all('td')
time = cols[0].text
high = float(cols[1].text)
low = float(cols[2].text)
data.append({'time': time, 'high': high, 'low': low})
```
4. 遍历分时数据,找出每日最高价和最低价的时间
```python
high_time = ''
high_value = 0
low_time = ''
low_value = float('inf')
for d in data:
if d['high'] > high_value:
high_value = d['high']
high_time = d['time']
if d['low'] < low_value:
low_value = d['low']
low_time = d['time']
print('最高价时间:', high_time)
print('最低价时间:', low_time)
```
5. 统计60%的最高价时间区间和最低价时间区间
```python
high_values = [d['high'] for d in data]
low_values = [d['low'] for d in data]
high_values.sort()
low_values.sort()
high_threshold = high_values[int(len(high_values) * 0.6)]
low_threshold = low_values[int(len(low_values) * 0.4)]
high_start = ''
high_end = ''
low_start = ''
low_end = ''
for d in data:
if d['high'] >= high_threshold and not high_start:
high_start = d['time']
if d['high'] < high_threshold and high_start and not high_end:
high_end = d['time']
if d['low'] <= low_threshold and not low_start:
low_start = d['time']
if d['low'] > low_threshold and low_start and not low_end:
low_end = d['time']
print('最高价时间区间:', high_start, '-', high_end)
print('最低价时间区间:', low_start, '-', low_end)
```
完整代码如下:
相关推荐
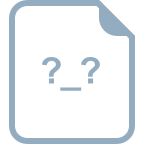
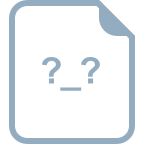














