像商城和超市这样的地方,都需要有自己的库房,并且库房商品的库存变化有专人记录,这样才能保证商城和超市正常运转。 本例要求编写一个程序,模拟库存管理系统。该系统主要包括系统首页、商品入库、商品显示和删除商品功能。每个功能的具体要求如下: (1) 系统的首页:用于显示系统所有的操作,并且可以选择使用某一个功能。 (2) 商品入库功能:首先提示是否要录入商品,根据用户输入的信息判断是否需要录入商品。如果需要录入商品,则需要用户输入商品的名称、颜色、价格和数量等信息。录入完成后,提示商品录入成功并打印所有商品。如果不需要录入商品,则返回系统首页。 (3) 商品显示功能:用户选择商品显示功能后,在控制台打印仓库所有商品信息。 (4) 删除商品功能:用户选择删除商品功能后,根据用户输入的商品编号删除商品,并在控制台打印删除后的所有商品。 本案例要求使用Collection集合存储自定义的对象,并用迭代器、增强for循环遍历集合。
时间: 2023-12-14 09:40:07 浏览: 41
好的,我来给您写一个Java程序实现这个需求。以下是代码实现及注释:
```java
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.Scanner;
public class InventoryManagementSystem {
static Collection<Commodity> inventory = new ArrayList<Commodity>(); //创建一个集合来存储商品信息
static Scanner scanner = new Scanner(System.in); //创建Scanner对象来读取用户输入
public static void main(String[] args) {
System.out.println("欢迎使用库存管理系统!");
while (true) {
System.out.println("请选择您要进行的操作:");
System.out.println("1. 商品入库");
System.out.println("2. 商品显示");
System.out.println("3. 删除商品");
System.out.println("4. 退出系统");
int choice = scanner.nextInt(); //读取用户输入的操作选项
switch (choice) {
case 1:
addCommodity();
break;
case 2:
showInventory();
break;
case 3:
deleteCommodity();
break;
case 4:
System.out.println("感谢使用库存管理系统,再见!");
System.exit(0); //退出程序
default:
System.out.println("无效的操作选项,请重新输入。");
break;
}
}
}
/**
* 商品类
*/
static class Commodity {
String name; //商品名称
String color; //商品颜色
double price; //商品价格
int quantity; //商品数量
public Commodity(String name, String color, double price, int quantity) {
this.name = name;
this.color = color;
this.price = price;
this.quantity = quantity;
}
@Override
public String toString() {
return "商品名称:" + name + ",商品颜色:" + color + ",商品价格:" + price + "元,商品数量:" + quantity + "件";
}
}
/**
* 商品入库
*/
static void addCommodity() {
System.out.println("您是否要录入商品?(Y/N)");
String input = scanner.next();
if (input.equalsIgnoreCase("Y")) {
System.out.println("请输入商品名称:");
String name = scanner.next();
System.out.println("请输入商品颜色:");
String color = scanner.next();
System.out.println("请输入商品价格:");
double price = scanner.nextDouble();
System.out.println("请输入商品数量:");
int quantity = scanner.nextInt();
Commodity commodity = new Commodity(name, color, price, quantity);
inventory.add(commodity); //将商品信息添加到集合中
System.out.println("商品录入成功!");
} else if (input.equalsIgnoreCase("N")) {
System.out.println("返回系统首页。");
} else {
System.out.println("无效的输入!");
}
}
/**
* 商品显示
*/
static void showInventory() {
System.out.println("仓库里的所有商品信息如下:");
Iterator<Commodity> iterator = inventory.iterator(); //创建迭代器遍历集合
while (iterator.hasNext()) {
Commodity commodity = iterator.next();
System.out.println(commodity.toString());
}
}
/**
* 删除商品
*/
static void deleteCommodity() {
System.out.println("请输入要删除的商品编号:");
int index = scanner.nextInt();
if (index > 0 && index <= inventory.size()) { //判断输入的商品编号是否有效
Iterator<Commodity> iterator = inventory.iterator(); //创建迭代器遍历集合
int i = 1;
while (iterator.hasNext()) {
iterator.next();
if (i == index) {
iterator.remove(); //从集合中删除指定商品信息
System.out.println("商品删除成功!");
break;
}
i++;
}
} else {
System.out.println("无效的商品编号!");
}
}
}
```
以上是一个简单的库存管理系统实现,欢迎您参考。
相关推荐
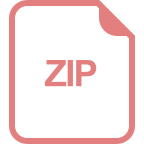















