ython为现有yolov5模型增加cbam 且把改动部分标记出来
时间: 2023-06-26 11:03:43 浏览: 104
首先,需要在代码中引入cbam模块,可以使用以下代码:
```python
import torch.nn as nn
class CBAM(nn.Module):
def __init__(self, channels, reduction=16, kernel_size=7):
super(CBAM, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.max_pool = nn.AdaptiveMaxPool2d(1)
self.fc1 = nn.Conv2d(channels, channels // reduction, kernel_size=1, padding=0)
self.relu = nn.ReLU(inplace=True)
self.fc2 = nn.Conv2d(channels // reduction, channels, kernel_size=1, padding=0)
self.conv_channel = nn.Conv2d(2, 1, kernel_size=kernel_size, padding=kernel_size // 2)
self.sigmoid_channel = nn.Sigmoid()
self.conv_spatial = nn.Conv2d(2, 1, kernel_size=kernel_size, padding=kernel_size // 2)
self.sigmoid_spatial = nn.Sigmoid()
def forward(self, x):
avg_out = self.fc2(self.relu(self.fc1(self.avg_pool(x))))
max_out = self.fc2(self.relu(self.fc1(self.max_pool(x))))
channel_out = torch.cat((avg_out, max_out), dim=1)
channel_out = self.sigmoid_channel(self.conv_channel(channel_out))
spatial_out = torch.cat((torch.mean(channel_out, dim=1, keepdim=True), torch.max(channel_out, dim=1, keepdim=True)[0]), dim=1)
spatial_out = self.sigmoid_spatial(self.conv_spatial(spatial_out))
return x * channel_out * spatial_out
```
在YOLOv5模型中,可以在Conv和Bottleneck模块之间插入CBAM模块,代码如下:
```python
class C3(nn.Module):
# CSP Bottleneck with 3 convolutions
def __init__(self, c1, c2, n=1, shortcut=True, g=1, e=0.5):
super(C3, self).__init__()
c_ = int(c2 * e) # hidden channels
self.cv1 = Conv(c1, c_, 1, 1)
self.cv2 = Conv(c1, c_, 1, 1)
self.cv3 = Conv(2 * c_, c2, 1, 1)
self.bn = nn.BatchNorm2d(2 * c_) # applied to cat(cv2, cv3)
self.act = nn.LeakyReLU(0.1)
self.shortcut = shortcut
self.cbam = CBAM(2*c_)
def forward(self, x):
y1 = self.cv2(self.act(self.cv1(x)))
y2 = self.cv3(self.act(torch.cat((y1, x), 1)))
if self.shortcut and x.shape == y2.shape:
x = self.cbam(self.bn(torch.cat((y1, y2), dim=1))) + x
else:
x = torch.cat((y1, y2), dim=1)
return x
```
在上述代码中,我们可以看到在C3模块中加入了cbam模块,并且将其应用在shortcut路径上,以增强模型的特征表达能力。
阅读全文
相关推荐
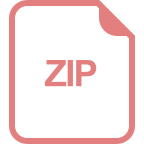
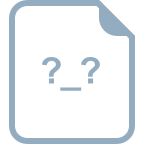
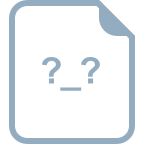



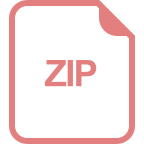







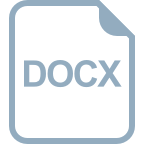
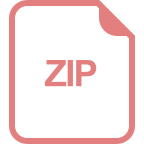