由pHash算法识别出的相似图片的GUI代码
时间: 2024-05-12 20:13:12 浏览: 8
以下是使用Python和PyQt5编写的简单GUI代码示例,用于使用pHash算法识别相似图片:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QVBoxLayout, QHBoxLayout, QPushButton, QFileDialog
from PyQt5.QtGui import QPixmap
import imagehash
from PIL import Image
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle('Image Similarity Checker')
# 创建组件
self.label1 = QLabel('Image 1:')
self.label2 = QLabel('Image 2:')
self.image1 = QLabel()
self.image2 = QLabel()
self.button1 = QPushButton('Upload Image 1')
self.button2 = QPushButton('Upload Image 2')
self.compare_button = QPushButton('Compare Images')
# 创建布局
self.layout = QVBoxLayout()
self.layout1 = QHBoxLayout()
self.layout2 = QHBoxLayout()
# 添加组件到布局中
self.layout1.addWidget(self.label1)
self.layout1.addWidget(self.image1)
self.layout1.addWidget(self.button1)
self.layout2.addWidget(self.label2)
self.layout2.addWidget(self.image2)
self.layout2.addWidget(self.button2)
self.layout.addLayout(self.layout1)
self.layout.addLayout(self.layout2)
self.layout.addWidget(self.compare_button)
# 设置主窗口布局
self.setLayout(self.layout)
# 连接按钮点击事件
self.button1.clicked.connect(self.upload_image1)
self.button2.clicked.connect(self.upload_image2)
self.compare_button.clicked.connect(self.compare_images)
self.image1_path = None
self.image2_path = None
# 上传图片1
def upload_image1(self):
fname = QFileDialog.getOpenFileName(self, 'Open file', './')
if fname[0]:
self.image1_path = fname[0]
pixmap = QPixmap(fname[0])
self.image1.setPixmap(pixmap)
# 上传图片2
def upload_image2(self):
fname = QFileDialog.getOpenFileName(self, 'Open file', './')
if fname[0]:
self.image2_path = fname[0]
pixmap = QPixmap(fname[0])
self.image2.setPixmap(pixmap)
# 比较图片
def compare_images(self):
if self.image1_path and self.image2_path:
hash1 = imagehash.average_hash(Image.open(self.image1_path))
hash2 = imagehash.average_hash(Image.open(self.image2_path))
similarity = hash1 - hash2
if similarity == 0:
message = 'The images are identical'
else:
message = f'The images are {similarity} bits different'
# 创建messagebox并显示结果
QMessageBox.information(self, 'Image Similarity', message)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
该程序使用PyQt5创建了一个简单的GUI,有两个上传按钮,用于选择要比较的两个图像,并有一个比较按钮。当用户单击“比较”按钮时,程序将使用pHash算法计算两个图像之间的相似度,并在消息框中显示相似度。
相关推荐
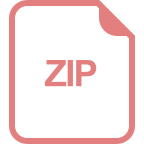
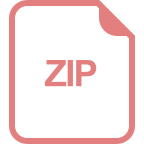














