在Android中用java写一个利用pHash算法,用来找出 List<File>中所有具有相同图片的图片的方法
时间: 2024-01-21 18:16:31 浏览: 26
pHash是一种图像指纹算法,可以将图像转化为一个64位的哈希值,因此可以利用这个哈希值来找出相同的图片。以下是一个示例代码,通过遍历List<File>中的所有文件,计算它们的pHash值,并将具有相同pHash值的文件分为一组。
```java
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.codec.digest.DigestUtils;
public class PHashFinder {
private static final int HASH_SIZE = 64;
// 计算pHash值
public static String getHash(File file) throws IOException {
String hash = "";
try {
BufferedImage img = ImageIO.read(file);
img = resize(img, 32, 32);
double[][] vals = new double[32][32];
for (int i = 0; i < 32; i++) {
for (int j = 0; j < 32; j++) {
vals[i][j] = getGray(img.getRGB(i, j));
}
}
double[][] dctVals = applyDCT(vals);
double total = 0;
double[] first8x8Vals = new double[HASH_SIZE];
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
first8x8Vals[i * 8 + j] = dctVals[i][j];
total += dctVals[i][j];
}
}
double avg = total / 64;
for (int i = 0; i < HASH_SIZE; i++) {
if (first8x8Vals[i] > avg) {
hash += "1";
} else {
hash += "0";
}
}
} catch (Exception e) {
e.printStackTrace();
}
return hash;
}
private static double getGray(int rgb) {
Color c = new Color(rgb);
return 0.299 * c.getRed() + 0.587 * c.getGreen() + 0.114 * c.getBlue();
}
private static double[][] applyDCT(double[][] f) {
int N = 32;
double[][] F = new double[N][N];
for (int u = 0; u < N; u++) {
for (int v = 0; v < N; v++) {
double sum = 0.0;
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
sum += Math.cos(((2 * i + 1) / (2.0 * N)) * u * Math.PI)
* Math.cos(((2 * j + 1) / (2.0 * N)) * v * Math.PI) * (f[i][j]);
}
}
sum *= ((u == 0) ? 1.0 / Math.sqrt(2) : 1.0) * ((v == 0) ? 1.0 / Math.sqrt(2) : 1.0)
/ Math.sqrt(2 * N);
F[u][v] = sum;
}
}
return F;
}
private static BufferedImage resize(BufferedImage image, int width, int height) {
BufferedImage resized = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g = resized.createGraphics();
g.drawImage(image, 0, 0, width, height, null);
g.dispose();
return resized;
}
// 找出具有相同pHash值的图片
public static Map<String, List<File>> findDuplicateImages(List<File> files) {
Map<String, List<File>> map = new HashMap<>();
for (File file : files) {
try {
String hash = getHash(file);
if (!map.containsKey(hash)) {
map.put(hash, new ArrayList<>());
}
map.get(hash).add(file);
} catch (IOException e) {
e.printStackTrace();
}
}
return map;
}
public static void main(String[] args) {
List<File> files = new ArrayList<>();
files.add(new File("/path/to/image1.jpg"));
files.add(new File("/path/to/image2.jpg"));
files.add(new File("/path/to/image3.jpg"));
files.add(new File("/path/to/image4.jpg"));
Map<String, List<File>> map = findDuplicateImages(files);
for (String hash : map.keySet()) {
List<File> duplicateFiles = map.get(hash);
if (duplicateFiles.size() > 1) {
System.out.println("Files with hash " + hash + ":");
for (File file : duplicateFiles) {
System.out.println(file.getAbsolutePath());
}
}
}
}
}
```
这段代码使用了Java的ImageIO库来读取图片文件,并计算它们的pHash值。然后将具有相同pHash值的文件分组,并输出结果。需要注意的是,这个示例代码仅适用于扩展名为jpg、jpeg、png和bmp的图片文件。如果需要处理其他类型的图片文件,则需要对代码进行适当的修改。
相关推荐
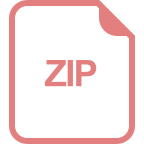
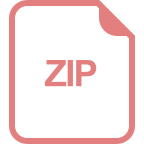














