写一个cpp算法函数, 支持QColor 转换为 QVector4D, QVector4D中的值区间为0-1
时间: 2023-05-26 14:02:32 浏览: 53
下面是一个cpp算法函数,支持将QColor 转换为 QVector4D,并且将QVector4D中的值区间限制在0-1之间:
```cpp
QVector4D colorToVector4D(const QColor& color) {
float r = color.red() / 255.0f;
float g = color.green() / 255.0f;
float b = color.blue() / 255.0f;
float a = color.alpha() / 255.0f;
return QVector4D(r, g, b, a).normalized();
}
```
首先将QColor中的RGBA值分别除以255.0f,将其范围限制在0-1之间。然后,使用QVector4D(r, g, b, a)构造函数创建一个四元组,并调用其normalized()方法将其标准化,确保其长度为1,未超出范围。最后,将QVector4D返回作为结果。
相关问题
写一个cpp算法函数, 支持QColor 转换为 QVector4D
#include <QVector4D>
#include <QColor>
QVector4D QColorToVector4D(const QColor& color)
{
QVector4D vector;
vector.setX(color.redF());
vector.setY(color.greenF());
vector.setZ(color.blueF());
vector.setW(color.alphaF());
return vector;
}
// 使用方法
QColor color(255, 0, 0, 127);
QVector4D vector = QColorToVector4D(color); // 将QColor转换为QVector4D
float red = vector.x(); // 获取红色分量
float green = vector.y(); // 获取绿色分量
float blue = vector.z(); // 获取蓝色分量
float alpha = vector.w(); // 获取透明度分量
在qt程序中,给我一个修改图片亮度和对比度的算法函数
好的,以下是一个简单的修改图片亮度和对比度的算法函数,使用 Qt 的 QImage 类实现:
```cpp
QImage adjustBrightnessContrast(const QImage& image, int brightness, int contrast)
{
QImage resultImage(image.size(), QImage::Format_ARGB32);
double contrastFactor = (259.0 * (contrast + 255.0)) / (255.0 * (259.0 - contrast));
double brightnessFactor = brightness / 255.0;
for(int y = 0; y < image.height(); y++)
{
for(int x = 0; x < image.width(); x++)
{
QColor color(image.pixel(x, y));
int red = qBound(0, int(contrastFactor * (color.red() - 128) + 128 + 255 * brightnessFactor), 255);
int green = qBound(0, int(contrastFactor * (color.green() - 128) + 128 + 255 * brightnessFactor), 255);
int blue = qBound(0, int(contrastFactor * (color.blue() - 128) + 128 + 255 * brightnessFactor), 255);
resultImage.setPixel(x, y, qRgb(red, green, blue));
}
}
return resultImage;
}
```
其中,brightness 表示亮度调整值(正值表示增加亮度,负值表示降低亮度),contrast 表示对比度调整值(正值表示增加对比度,负值表示降低对比度)。函数会返回一个调整过亮度和对比度的 QImage 对象。
相关推荐
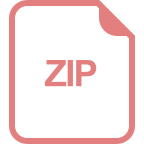












