QCustomPlot* TypeCustom = new QCustomPlot(); TypeCustom = ui->widget_type_curve; TypeCustom->legend->setVisible(true); TypeCustom->legend->setFont(QFont("Helvetica", 9)); QPen pen; QStringList lineNames; lineNames << "lsNone" << "lsLine" << "lsStepLeft" << "lsStepRight" << "lsStepCenter" << "lsImpulse"; TypeCustom->addGraph(); pen.setColor(QColor(qSin(4*1+1.2)*80+80, qSin(4*0.3+0)*80+80, qSin(4*0.3+1.5)*80+80)); TypeCustom->graph()->setPen(pen); TypeCustom->graph()->setName("123"); TypeCustom->graph()->setLineStyle((QCPGraph::LineStyle)4); TypeCustom->graph()->setScatterStyle(QCPScatterStyle(QCPScatterStyle::ssCircle, 5)); // generate data: QVector<double> x(15), y(15); for (int j=0; j<15; ++j) { x[j] = j/15.0 * 5*3.14 + 0.01; y[j] = 7*qSin(x[j])/x[j] - (4-QCPGraph::lsNone)*5 + (QCPGraph::lsImpulse)*5 + 2; } TypeCustom->graph()->setData(x, y); TypeCustom->graph()->rescaleAxes(true); // zoom out a bit: TypeCustom->yAxis->scaleRange(1.1, TypeCustom->yAxis->range().center()); TypeCustom->xAxis->scaleRange(1.1, TypeCustom->xAxis->range().center()); // set blank axis lines: TypeCustom->xAxis->setTicks(false); TypeCustom->yAxis->setTicks(true); TypeCustom->xAxis->setTickLabels(false); TypeCustom->yAxis->setTickLabels(true); // make top right axes clones of bottom left axes: TypeCustom->axisRect()->setupFullAxesBox(); 给我解释一下上述代码
时间: 2024-04-18 19:24:08 浏览: 198
上述代码是一个使用QCustomPlot库创建和配置一个定义曲线图的示例。下面是对代码的解释:
1. 创建一个QCustomPlot对象,并将其设置为ui中的widget_type_curve部件的指针:
```cpp
QCustomPlot* TypeCustom = new QCustomPlot();
TypeCustom = ui->widget_type_curve;
```
2. 设置图例可见,并设置其字体为Helvetica字体大小为9:
```cpp
TypeCustom->legend->setVisible(true);
TypeCustom->legend->setFont(QFont("Helvetica", 9));
```
3. 创建一个QPen对象,并设置线条名称的字符串列表:
```cpp
QPen pen;
QStringList lineNames;
lineNames << "lsNone" << "lsLine" << "lsStepLeft" << "lsStepRight" << "lsStepCenter" << "lsImpulse";
```
4. 添加一个曲线图(graph)到QCustomPlot对象中,并设置曲线的颜色、名称、线条样式和散点样式:
```cpp
TypeCustom->addGraph();
pen.setColor(QColor(qSin(4*1+1.2)*80+80, qSin(4*0.3+0)*80+80, qSin(4*0.3+1.5)*80+80));
TypeCustom->graph()->setPen(pen);
TypeCustom->graph()->setName("123");
TypeCustom->graph()->setLineStyle((QCPGraph::LineStyle)4);
TypeCustom->graph()->setScatterStyle(QCPScatterStyle(QCPScatterStyle::ssCircle, 5));
```
5. 生成数据并将其设置到曲线图中:
```cpp
QVector<double> x(15), y(15);
for (int j=0; j<15; ++j)
{
x[j] = j/15.0 * 5*3.14 + 0.01;
y[j] = 7*qSin(x[j])/x[j] - (4-QCPGraph::lsNone)*5 + (QCPGraph::lsImpulse)*5 + 2;
}
TypeCustom->graph()->setData(x, y);
```
6. 调整曲线图的坐标轴范围和刻度:
```cpp
TypeCustom->graph()->rescaleAxes(true);
TypeCustom->yAxis->scaleRange(1.1, TypeCustom->yAxis->range().center());
TypeCustom->xAxis->scaleRange(1.1, TypeCustom->xAxis->range().center());
```
7. 设置坐标轴的显示方式,其中x轴不显示刻度和标签,y轴显示刻度和标签:
```cpp
TypeCustom->xAxis->setTicks(false);
TypeCustom->yAxis->setTicks(true);
TypeCustom->xAxis->setTickLabels(false);
TypeCustom->yAxis->setTickLabels(true);
```
8. 将右上角坐标轴设置为与左下角坐标轴相同:
```cpp
TypeCustom->axisRect()->setupFullAxesBox();
```
这段代码的作用是创建一个自定义曲线图,并对其进行一系列的配置和设置,最终在ui中的widget_type_curve部件上显示出来。
阅读全文
相关推荐
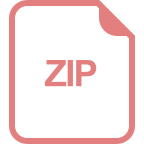
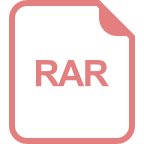
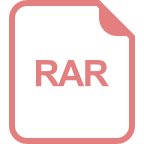















