c# File.ReadAllLines 方法使用 GB2312格式
时间: 2024-02-24 10:53:57 浏览: 22
可以使用以下代码来读取 GB2312 格式的文本文件:
```
string[] lines = File.ReadAllLines("file.txt", Encoding.GetEncoding("GB2312"));
```
其中,"file.txt" 是你要读取的文件路径。使用 Encoding.GetEncoding("GB2312") 指定编码格式为 GB2312。这样就可以读取 GB2312 格式的文本文件了。
相关问题
C# File.ReadAllText读中文乱码
C#中使用File.ReadAllText读取中文文件可能会出现乱码的情况。这是因为File.ReadAllText默认使用UTF-8编码读取文件,而如果文件的编码不是UTF-8,就会出现中文乱码的情况。
解决方法有两种:
1.指定文件编码
可以通过指定文件编码来解决中文乱码的问题。例如,如果文件编码为GB2312,可以这样读取文件:
```
string content = File.ReadAllText(filepath, Encoding.GetEncoding("GB2312"));
```
2.使用StreamReader读取文件
使用StreamReader类可以更加灵活地读取文件,并且可以指定文件编码。例如,可以这样读取文件:
```
using (StreamReader sr = new StreamReader(filepath, Encoding.GetEncoding("GB2312")))
{
string content = sr.ReadToEnd();
}
```
以上两种方法都可以解决中文乱码的问题,具体选择哪一种方法取决于具体情况。
C#File.move
你好!C#中的`File.Move`方法用于将一个文件移动到指定的位置。它接受两个参数,第一个参数是要移动的文件的路径和名称,第二个参数是目标位置的路径和名称。
以下是一个示例代码,演示如何使用`File.Move`方法来移动文件:
```csharp
using System;
using System.IO;
class Program
{
static void Main()
{
string sourceFilePath = "C:\\path\\to\\source\\file.txt";
string destinationFilePath = "C:\\path\\to\\destination\\file.txt";
try
{
// 移动文件
File.Move(sourceFilePath, destinationFilePath);
Console.WriteLine("文件移动成功!");
}
catch (IOException ex)
{
Console.WriteLine("发生错误:" + ex.Message);
}
}
}
```
在上面的示例中,我们指定了要移动的源文件路径和目标文件路径。然后,使用`File.Move`方法将源文件移动到目标位置。如果移动成功,将输出"文件移动成功!";如果发生错误,将输出错误消息。
希望这可以帮助到你!如果你有更多问题,请随时问我。
相关推荐
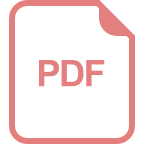
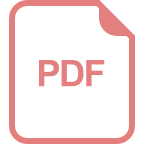
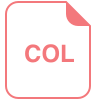











