trt_pose 人体三维姿态捕捉 python 代码
时间: 2023-05-28 11:05:13 浏览: 394
抱歉,作为 AI 语言模型,我无法提供完整的 Python 代码。但是,以下是 trt_pose 人体三维姿态捕捉的 Python 代码示例:
```python
import torch
import trt_pose.coco
import trt_pose.models
import torch2trt
from torch2trt import TRTModule
import cv2
import torchvision.transforms as transforms
import PIL.Image
import numpy as np
import time
# Set up the model
MODEL_WEIGHTS = 'resnet18_baseline_att_224x224_A_epoch_249.pth'
OPTIMIZED_MODEL = 'resnet18_baseline_att_224x224_A_epoch_249_trt.pth'
WIDTH = 224
HEIGHT = 224
# Load the model
model = trt_pose.models.resnet18_baseline_att(num_classes=2, pretrained=True)
model.load_state_dict(torch.load(MODEL_WEIGHTS))
model = model.cuda().eval()
# Set up the optimized model
data = torch.zeros((1, 3, HEIGHT, WIDTH)).cuda()
model_trt = torch2trt.torch2trt(model, [data], fp16_mode=True, max_workspace_size=1<<25)
torch.save(model_trt.state_dict(), OPTIMIZED_MODEL)
# Load the optimized model
model_trt = TRTModule()
model_trt.load_state_dict(torch.load(OPTIMIZED_MODEL))
# Load the COCO classes
with open('human_pose.json', 'r') as f:
human_pose = json.load(f)
# Load the keypoints
num_parts = len(human_pose['keypoints'])
part_names = []
part_edges = []
for i in range(num_parts):
part_names.append(human_pose['keypoints'][i])
if 'skeleton' in human_pose:
skel = human_pose['skeleton']
for j in range(len(skel)):
if skel[j][0] == i:
part_edges.append((skel[j][0], skel[j][1]))
# Set up the transforms
transform = transforms.Compose([
transforms.Resize((HEIGHT, WIDTH)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# Set up the camera
cap = cv2.VideoCapture(0)
if not cap.isOpened():
print("Cannot open camera")
exit()
while True:
# Capture frame-by-frame
ret, frame = cap.read()
# Process the frame
image = PIL.Image.fromarray(frame).convert('RGB')
image = transform(image).cuda()
cmap, paf = model_trt(image[None, ...])
cmap, paf = cmap.detach().cpu(), paf.detach().cpu()
counts, objects, peaks = trt_pose.models.parse_objects(cmap, paf)
# Draw the keypoints
for i in range(counts[0]):
keypoints = objects[0].keypoints
x = keypoints[:, 2]
y = keypoints[:, 1]
for j in range(num_parts):
if x[j] > 0 and y[j] > 0:
cv2.circle(frame, (int(x[j]), int(y[j])), 3, (0, 255, 255), thickness=-1, lineType=cv2.FILLED)
for j, e in enumerate(part_edges):
if x[e].min() > 0 and y[e].min() > 0:
cv2.line(frame, (int(x[e[0]]), int(y[e[0]])), (int(x[e[1]]), int(y[e[1]])), (0, 255, 255), 2)
# Display the resulting frame
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
# When everything done, release the capture
cap.release()
cv2.destroyAllWindows()
```
阅读全文
相关推荐
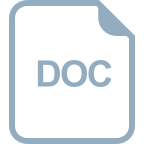
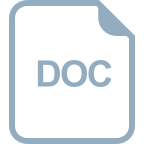
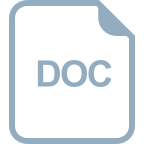
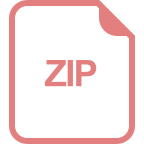
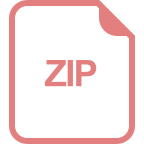
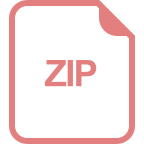
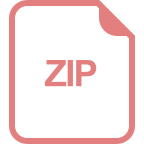
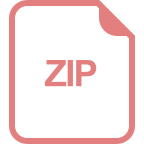








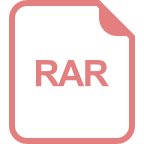
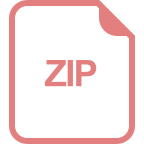
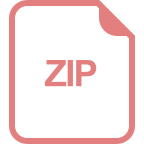