C语言:编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其他 字符的个数,在主函数中输入字符串以及输出上述结果。不用全局变量
时间: 2023-11-17 17:02:05 浏览: 50
以下是一个实现此功能的 C 语言函数:
```c
#include <stdio.h>
#include <ctype.h>
void count_char_types(char *str, int *alpha_count, int *digit_count, int *space_count, int *other_count) {
// 初始化各个计数器
*alpha_count = *digit_count = *space_count = *other_count = 0;
// 遍历字符串中的每个字符,分别统计字母、数字、空格和其他字符的个数
while (*str != '\0') {
if (isalpha(*str)) {
(*alpha_count)++;
} else if (isdigit(*str)) {
(*digit_count)++;
} else if (isspace(*str)) {
(*space_count)++;
} else {
(*other_count)++;
}
str++;
}
}
int main() {
char str[100];
int alpha_count, digit_count, space_count, other_count;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
count_char_types(str, &alpha_count, &digit_count, &space_count, &other_count);
printf("字母个数:%d\n", alpha_count);
printf("数字个数:%d\n", digit_count);
printf("空格个数:%d\n", space_count);
printf("其他字符个数:%d\n", other_count);
return 0;
}
```
在主函数中,我们先定义一个长度为 100 的字符数组 `str`,用于存储用户输入的字符串。然后,我们调用 `count_char_types` 函数,将字符串和各个计数器的地址作为实参传递给该函数。在 `count_char_types` 函数中,我们使用 `while` 循环遍历字符串中的每个字符,根据字符的类型分别增加相应的计数器。最后,我们在主函数中输出各个计数器的值即可。
需要注意的是,在调用 `fgets` 函数读取用户输入的字符串时,我们需要指定读取字符串的最大长度,以避免出现缓冲区溢出的情况。
相关推荐
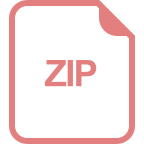
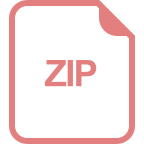
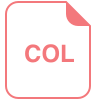
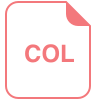
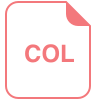
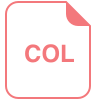
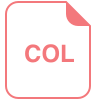









