C++编写一个员工类包含名字,员工号和工资,再从员工类派生出销售人员,工人,行政人员,分别包含成员变量,每月销售产品数量,每月生产产品数量和工资时间,要求各类中都有成员函数pay(),用来计算每个人的月薪,假设每件产品利润200,行政人员每小时工作酬金50,规定销售人员工资为每月销售利润的百分之二十,工人工资为每月生产产品乘以每件利润的百分之十,行政人员的工资为每月工作时间乘以每月工作时间)
时间: 2023-12-15 14:03:32 浏览: 122
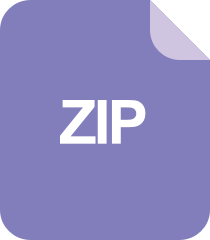
员工工资管理 C++

好的,以下是员工类和三个派生类的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Employee {
private:
string name;
int id;
double salary;
public:
Employee(string n, int i, double s) : name(n), id(i), salary(s) {}
virtual double pay() { return salary; }
};
class Salesman : public Employee {
private:
int sales;
public:
Salesman(string n, int i, double s, int sls) : Employee(n, i, s), sales(sls) {}
virtual double pay() { return salary + sales * 200 * 0.2; }
};
class Worker : public Employee {
private:
int production;
public:
Worker(string n, int i, double s, int p) : Employee(n, i, s), production(p) {}
virtual double pay() { return salary + production * 200 * 0.1; }
};
class Administrator : public Employee {
private:
int worktime;
public:
Administrator(string n, int i, double s, int w) : Employee(n, i, s), worktime(w) {}
virtual double pay() { return salary + worktime * worktime * 50; }
};
int main() {
Employee* e1 = new Salesman("Tom", 1001, 3000, 100);
Employee* e2 = new Worker("Jerry", 1002, 2500, 200);
Employee* e3 = new Administrator("Alice", 1003, 4000, 160);
cout << e1->pay() << endl;
cout << e2->pay() << endl;
cout << e3->pay() << endl;
delete e1;
delete e2;
delete e3;
return 0;
}
```
上述代码中,Employee 是基类,包含三个私有成员变量:姓名、员工号和工资。其中,构造函数用来进行初始化。还有一个虚函数 pay(),用来计算每个人的月薪。派生类 Salesman、Worker、Administrator 是从 Employee 派生而来,分别代表销售人员、工人和行政人员。这三个类分别新增了一个成员变量,分别是每月销售产品数量、每月生产产品数量和工资时间。这三个类都重载了 pay() 函数,用来计算每个人的月薪。
在主函数中,我们分别创建了三个对象,分别代表销售人员、工人和行政人员。然后通过基类指针调用 pay() 函数,输出每个人的月薪。最后,我们通过 delete 关键字释放了动态分配的内存。
阅读全文
相关推荐
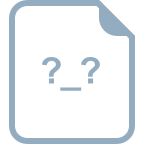
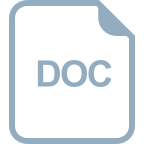
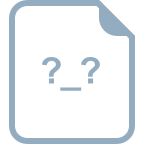
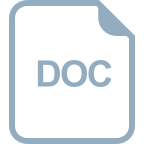


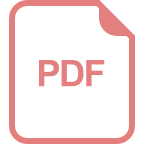
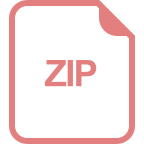
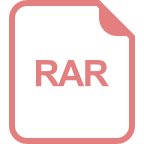
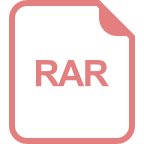
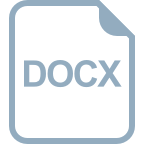
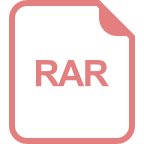
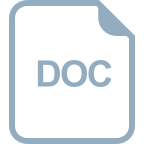