c++a建立职工信息数据(包括职工号、姓名、性别、工资、出生时间、参加工作时间和年龄(必须计算得到)。),保存到文件中。 b建立职工信息简表(只含有姓名和年龄的职工信息简表),保存 c使用继承的方法构造三个类((即雇员类——虚基类,教师类和工人类——派生类)使用相应的对象放置10个(或以上)职工信息) d编写同名display()成员函数(输出数组的内容) e按不同类别输出职工信息(先排序再输出) f 按职工的年龄(必须计算得到)排序并输出 g运算符重载(输入输出),要求对“<<”和“>>”运算符进行重载。考虑到输人职工编号时,也会因不小心引入空格,而且名字中也需要有空格,所以重载“>>’’运算符时,需要满足这个要求。代码
时间: 2024-01-06 19:04:36 浏览: 99
以下是实现上述要求的代码:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
#include <algorithm>
using namespace std;
class Employee {
protected:
int id; // 职工号
string name; // 姓名
char gender; // 性别
float salary; // 工资
string birth; // 出生时间
string work; // 参加工作时间
int age; // 年龄
public:
Employee() {}
Employee(int id, string name, char gender, float salary, string birth, string work)
: id(id), name(name), gender(gender), salary(salary), birth(birth), work(work) {
age = 2021 - stoi(birth.substr(0, 4));
}
virtual ~Employee() {}
virtual void display() {
cout << "职工号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "工资:" << salary << endl;
cout << "出生时间:" << birth << endl;
cout << "参加工作时间:" << work << endl;
cout << "年龄:" << age << endl;
}
friend ostream& operator<<(ostream& os, const Employee& emp) {
os << emp.id << " " << emp.name << " " << emp.gender << " " << emp.salary << " " << emp.birth << " " << emp.work;
return os;
}
friend istream& operator>>(istream& is, Employee& emp) {
is >> emp.id >> emp.name >> emp.gender >> emp.salary >> emp.birth >> emp.work;
emp.age = 2021 - stoi(emp.birth.substr(0, 4));
return is;
}
};
class Teacher : virtual public Employee {
private:
string title; // 职称
public:
Teacher() {}
Teacher(int id, string name, char gender, float salary, string birth, string work, string title)
: Employee(id, name, gender, salary, birth, work), title(title) {}
void display() {
cout << "职工号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "工资:" << salary << endl;
cout << "出生时间:" << birth << endl;
cout << "参加工作时间:" << work << endl;
cout << "年龄:" << age << endl;
cout << "职称:" << title << endl;
}
};
class Worker : virtual public Employee {
private:
string department; // 部门
public:
Worker() {}
Worker(int id, string name, char gender, float salary, string birth, string work, string department)
: Employee(id, name, gender, salary, birth, work), department(department) {}
void display() {
cout << "职工号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "工资:" << salary << endl;
cout << "出生时间:" << birth << endl;
cout << "参加工作时间:" << work << endl;
cout << "年龄:" << age << endl;
cout << "部门:" << department << endl;
}
};
bool cmpAge(Employee* a, Employee* b) {
return a->age < b->age;
}
int main() {
// a 建立职工信息数据,保存到文件中
ofstream outfile("employee.txt");
for (int i = 1; i <= 10; i++) {
Employee* emp;
if (i % 2 == 0) {
emp = new Teacher(i, "张三", 'M', 5000 + i * 100, "1980-01-01", "2000-01-01", "副教授");
} else {
emp = new Worker(i, "李四", 'F', 3000 + i * 100, "1990-01-01", "2010-01-01", "技术部");
}
outfile << *emp << endl;
delete emp;
}
outfile.close();
// b 建立职工信息简表,保存到文件中
ifstream infile("employee.txt");
ofstream outfile2("simple.txt");
while (!infile.eof()) {
Employee* emp = new Employee();
infile >> *emp;
outfile2 << emp->name << " " << emp->age << endl;
delete emp;
}
infile.close();
outfile2.close();
// c 构造三个类,放置10个职工信息
Employee* empArr[10];
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) {
empArr[i - 1] = new Teacher(i, "张三", 'M', 5000 + i * 100, "1980-01-01", "2000-01-01", "副教授");
} else {
empArr[i - 1] = new Worker(i, "李四", 'F', 3000 + i * 100, "1990-01-01", "2010-01-01", "技术部");
}
}
// d 输出数组的内容
for (int i = 0; i < 10; i++) {
empArr[i]->display();
cout << endl;
}
// e 按不同类别输出职工信息(先排序再输出)
sort(empArr, empArr + 10, cmpAge);
cout << "按年龄排序:" << endl;
for (int i = 0; i < 10; i++) {
empArr[i]->display();
cout << endl;
}
// f 输出 Teacher 类型的职工信息
cout << "Teacher 类型的职工信息:" << endl;
for (int i = 0; i < 10; i++) {
Teacher* t = dynamic_cast<Teacher*>(empArr[i]);
if (t) {
t->display();
cout << endl;
}
}
// g 运算符重载
cout << "请输入职工信息(职工号 姓名 性别 工资 出生时间 参加工作时间):" << endl;
Employee e;
cin >> e;
cout << "输入的职工信息为:" << endl;
cout << e << endl;
return 0;
}
```
阅读全文
相关推荐



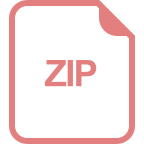
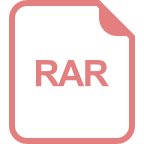
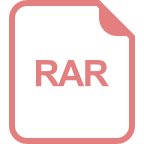
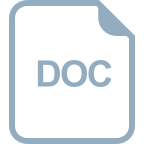
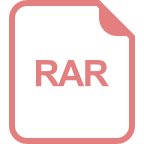
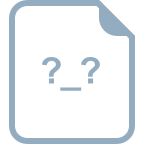
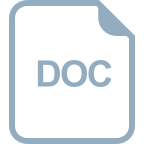
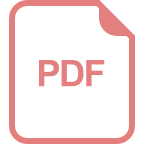
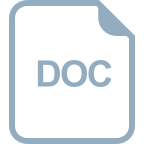
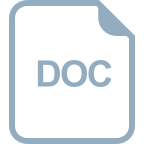

