编写Java应用程序,创建一个可序列化类,将该类的对象写入到文件中。用对象输入流读取并显示对象信息。写出完整的java代码
时间: 2024-03-19 10:44:25 浏览: 61
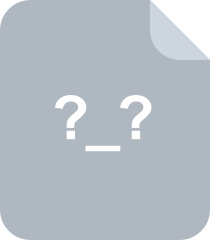
编写一个java应用程序
好的,以下是完整的 Java 代码,包括序列化和反序列化:
```java
import java.io.*;
public class SerializableDemo {
public static void main(String[] args) {
// Serialize object
Person person = new Person("Tom", 20);
try {
FileOutputStream fileOut = new FileOutputStream("person.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(person);
out.close();
fileOut.close();
System.out.println("Serialized data is saved in person.ser");
} catch (IOException i) {
i.printStackTrace();
}
// Deserialize object
Person deserializedPerson = null;
try {
FileInputStream fileIn = new FileInputStream("person.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
deserializedPerson = (Person) in.readObject();
in.close();
fileIn.close();
} catch (IOException i) {
i.printStackTrace();
} catch (ClassNotFoundException c) {
System.out.println("Person class not found");
c.printStackTrace();
}
System.out.println("Deserialized Person:");
System.out.println("Name: " + deserializedPerson.getName());
System.out.println("Age: " + deserializedPerson.getAge());
}
}
class Person implements Serializable {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
在该示例中,我们创建了一个 `Person` 类,并将其序列化为 `person.ser` 文件。然后,我们使用对象输入流读取该文件并反序列化 `Person` 对象,最后输出对象的属性值。
阅读全文
相关推荐
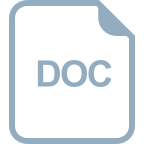





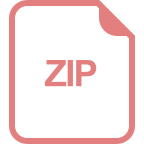
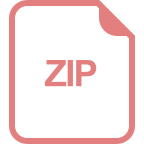
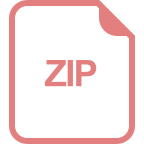
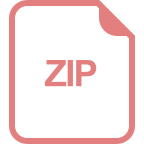
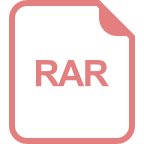
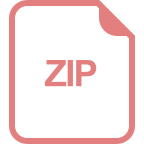
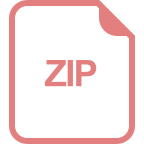
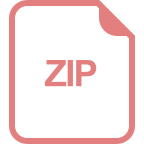
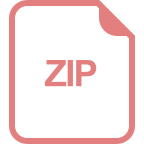
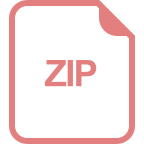