#include <bits/stdc++.h> using namespace std; class Box(){ public: Box(height=10;width=10;length=10); Box(int h,int w,int len):height(h),width(w),length(len){} void volume(); private: int height,width,length; }; void Box::volume(){ cout<<(height*width*length); } int main(){ Box box1; cout<<"The volume of box1 is"<<box1.volume()<<endl; Box box2(15,34,67); cout<<"The volume of box1 is"<<box2.volume()<<endl; return 0; }这个代码有什么问题
时间: 2024-01-17 11:04:39 浏览: 19
这个代码有以下问题:
1. 类定义中的构造函数声明中应该在形参前加上类型,如 Box(int height = 10, int width = 10, int length = 10)。
2. 成员函数 volume 应该返回一个值,而不是 void。
3. 在输出盒子体积时,应该输出换行符,以便更加清晰。
修改后的代码如下:
```
#include <bits/stdc++.h>
using namespace std;
class Box {
public:
Box(int height = 10, int width = 10, int length = 10);
Box(int h, int w, int len): height(h), width(w), length(len) {}
int volume();
private:
int height, width, length;
};
Box::Box(int height, int width, int length)
: height(height), width(width), length(length) {}
int Box::volume() {
return height * width * length;
}
int main() {
Box box1;
cout << "The volume of box1 is " << box1.volume() << endl;
Box box2(15, 34, 67);
cout << "The volume of box2 is " << box2.volume() << endl;
return 0;
}
```
相关推荐
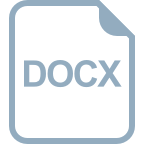
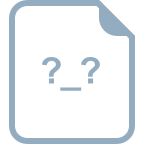
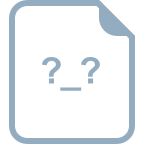















