平方乘算法java实现详细解析
时间: 2023-11-28 19:04:57 浏览: 35
平方乘算法是一种快速计算大数幂的算法,它的基本思想是利用指数的二进制分解和平方运算的性质,将指数的幂运算转化为若干个平方运算和乘法运算的组合,从而减少计算量。下面是平方乘算法的Java实现详细解析:
1.首先定义一个方法来实现平方乘算法,该方法接受三个参数:底数base、指数exponent和模数modulus,返回计算结果result。其中,底数、指数和模数都是大数,使用Java的BigInteger类来表示。
```java
public static BigInteger squareAndMultiply(BigInteger base, BigInteger exponent, BigInteger modulus) {
// TODO: 实现平方乘算法
return result;
}
```
2.将指数exponent转化为二进制形式,并保存在一个数组中。代码如下:
```java
String binaryExponent = exponent.toString(2);
int[] binaryArray = new int[binaryExponent.length()];
for (int i = 0; i < binaryExponent.length(); i++) {
binaryArray[i] = Integer.parseInt(String.valueOf(binaryExponent.charAt(i)));
}
```
3.初始化结果result为1,接着开始循环计算。
4.从数组的最高位开始,判断该位的值是否为1,如果为1,则将结果result乘以底数base,并对结果取模。
```java
if (binaryArray[i] == 1) {
result = result.multiply(base).mod(modulus);
}
```
5.将底数base平方,并对结果取模,以便在下一次循环中使用。
```java
base = base.multiply(base).mod(modulus);
```
6.循环结束后,返回最终的计算结果result。
完整的平方乘算法Java实现代码如下:
```java
public static BigInteger squareAndMultiply(BigInteger base, BigInteger exponent, BigInteger modulus) {
String binaryExponent = exponent.toString(2);
int[] binaryArray = new int[binaryExponent.length()];
for (int i = 0; i < binaryExponent.length(); i++) {
binaryArray[i] = Integer.parseInt(String.valueOf(binaryExponent.charAt(i)));
}
BigInteger result = BigInteger.ONE;
for (int i = binaryArray.length - 1; i >= 0; i--) {
if (binaryArray[i] == 1) {
result = result.multiply(base).mod(modulus);
}
base = base.multiply(base).mod(modulus);
}
return result;
}
```
使用该方法可以快速计算大数幂,例如计算2的100次方对10007取模的结果:
```java
BigInteger base = BigInteger.valueOf(2);
BigInteger exponent = BigInteger.valueOf(100);
BigInteger modulus = BigInteger.valueOf(10007);
BigInteger result = squareAndMultiply(base, exponent, modulus);
System.out.println(result); // 输出:5879
```
相关推荐
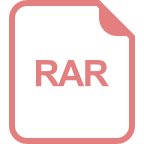
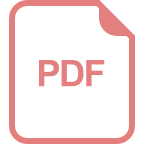














