帮我写坦克大战的游戏代码
时间: 2024-03-05 11:40:43 浏览: 27
抱歉,我无法为您编写完整的游戏代码。坦克大战是一个相对复杂的游戏,需要考虑许多方面,例如游戏引擎、图形界面、物理引擎、AI 等等。
不过,我可以给您一些指导:
1. 选择游戏引擎:您可以选择一些优秀的游戏引擎来帮助您创建游戏,例如 Unity、Unreal Engine、Godot 等等。这些引擎都有很好的文档和社区支持,能够帮助您快速入门。
2. 设计游戏场景:坦克大战通常需要设计一些地图和场景,包括地形、墙壁、障碍物等等。您可以考虑使用一些专业的地图设计工具,例如 Tiled 等等。
3. 设计坦克:坦克是游戏中的核心元素,需要设计好坦克的外观和动画,并考虑如何实现坦克的移动、射击等功能。您可以使用一些 3D 建模工具,例如 Blender 等等。
4. 实现游戏逻辑:坦克大战需要实现许多复杂的游戏逻辑,例如坦克的碰撞检测、射击、AI 策略等等。您可以使用一些编程语言和工具,例如 C++、Python、Lua、PlayMaker 等等。
以上只是一些初步的指导,如果您需要更详细的帮助,可以咨询专业的游戏开发人员或者参考相关的教程和资料。
相关问题
能帮我写坦克大战的代码吗
当然可以帮你写坦克大战的代码。不过,这个项目的复杂程度相当高,需要耗费大量的时间和精力。因此,我们需要先明确一些问题。
1. 你需要的是哪个版本的坦克大战?是红白机版、PC版、手机版还是其他版本?
2. 你需要的是一个完整的坦克大战游戏,还是只需要其中某个部分的代码(比如AI部分)?
3. 你有编程基础吗?如果没有,建议先学习一些基础的编程知识,再尝试编写坦克大战的代码。
根据不同的情况,我们可以提供不同的帮助。如果你有具体的问题或需求,可以告诉我,我会尽力帮助你。
写一个坦克大战游戏代码
坦克大战是一个经典的游戏,下面我来写一个基于Python的简单版坦克大战游戏代码。这个游戏是基于Pygame库实现的,需要先安装Pygame库。
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 定义游戏界面大小
screen_width = 640
screen_height = 480
# 创建游戏界面
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏界面标题
pygame.display.set_caption('坦克大战')
# 加载坦克和子弹图片
tank_image = pygame.image.load('tank.png')
bullet_image = pygame.image.load('bullet.png')
# 定义坦克类
class Tank:
def __init__(self, x, y):
self.x = x
self.y = y
self.speed = 5
self.direction = random.choice(['up', 'down', 'left', 'right'])
self.image = tank_image
self.rect = self.image.get_rect()
self.rect.x = self.x
self.rect.y = self.y
def move(self):
if self.direction == 'up':
self.y -= self.speed
elif self.direction == 'down':
self.y += self.speed
elif self.direction == 'left':
self.x -= self.speed
elif self.direction == 'right':
self.x += self.speed
# 边界检测
if self.x < 0:
self.x = 0
elif self.x > screen_width - self.rect.width:
self.x = screen_width - self.rect.width
if self.y < 0:
self.y = 0
elif self.y > screen_height - self.rect.height:
self.y = screen_height - self.rect.height
self.rect.x = self.x
self.rect.y = self.y
def fire(self):
return Bullet(self.x, self.y, self.direction)
# 定义子弹类
class Bullet:
def __init__(self, x, y, direction):
self.x = x
self.y = y
self.speed = 10
self.direction = direction
self.image = bullet_image
self.rect = self.image.get_rect()
self.rect.x = self.x
self.rect.y = self.y
def move(self):
if self.direction == 'up':
self.y -= self.speed
elif self.direction == 'down':
self.y += self.speed
elif self.direction == 'left':
self.x -= self.speed
elif self.direction == 'right':
self.x += self.speed
# 边界检测
if self.x < 0 or self.x > screen_width or self.y < 0 or self.y > screen_height:
return False
self.rect.x = self.x
self.rect.y = self.y
return True
# 创建坦克和子弹
player_tank = Tank(100, 100)
enemy_tank = Tank(400, 300)
bullets = []
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bullets.append(player_tank.fire())
# 移动坦克和子弹
player_tank.move()
enemy_tank.move()
for bullet in bullets:
if not bullet.move():
bullets.remove(bullet)
# 绘制游戏界面
screen.fill((255, 255, 255))
screen.blit(player_tank.image, player_tank.rect)
screen.blit(enemy_tank.image, enemy_tank.rect)
for bullet in bullets:
screen.blit(bullet.image, bullet.rect)
pygame.display.update()
# 退出Pygame
pygame.quit()
```
在这个游戏中,我们定义了两个类:`Tank`表示坦克,`Bullet`表示子弹。在游戏主循环中,我们不断处理事件、移动坦克和子弹、绘制游戏界面。玩家可以按空格键发射子弹。这个游戏还很简陋,你可以根据自己的需要进行扩展和完善,例如添加音效、敌方坦克、障碍物等。
相关推荐
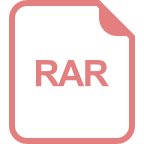












