Bird类实现了Flyer接口。 改变Start类的主要方法来测试喜鹊、鸵鸟和pegasi 界面。
时间: 2024-05-01 11:18:51 浏览: 204
对于这个问题,我可以回答:可以通过在Start类的主要方法中分别实例化喜鹊、鸵鸟和pegasi,并调用它们实现的Flyer接口中的方法来测试它们的飞行能力。具体实现可以参考以下代码:
```
public class Start {
public static void main(String[] args) {
Flyer[] flyers = {new Magpie(), new Ostrich(), new Pegasus()};
for (Flyer flyer : flyers) {
flyer.fly();
}
}
}
interface Flyer {
void fly();
}
class Bird {
}
class Magpie extends Bird implements Flyer {
@Override
public void fly() {
System.out.println("喜鹊在飞行。");
}
}
class Ostrich extends Bird {
}
class Pegasus implements Flyer {
@Override
public void fly() {
System.out.println("飞马在飞行。");
}
}
```
希望这个回答对您有帮助。
相关问题
用Java书写Bird类实现了Flyer接口。 当通过Flyer界面查看时,更改Start类的main方法来测试magpies、ostriches和pegasi。
好的,这是一个关于Java编程的问题,我可以回答。假设Flyer界面包含了fly()方法,Bird类实现了Flyer接口,则代码可能如下所示:
public interface Flyer {
void fly();
}
public class Bird implements Flyer {
public void fly() {
// 飞的具体实现
}
}
在Start类的main方法中,我们可以创建magpies、ostriches和pegasi对象,并调用fly()方法来测试它们是否能飞行:
public static void main(String[] args) {
Flyer magpies = new Bird();
Flyer ostriches = new Bird();
Flyer pegasi = new Bird();
magpies.fly();
ostriches.fly();
pegasi.fly();
}
希望这能回答您的问题。
定义接口flyer 定义接口runner定义类bird implements flyer ,runner定义测试类测试bird的能力
在面向对象编程中,接口是一种契约,它定义了一组规范,允许类去实现并提供这些功能。假设我们有两个接口,`Flyer` 和 `Runner`,分别代表能够飞翔和奔跑的行为:
```java
// 定义接口 Flyer
interface Flyer {
void fly();
}
// 定义接口 Runner
interface Runner {
void run();
}
```
然后我们创建一个名为 `Bird` 的类,它实现了这两个接口:
```java
// 定义类 Bird 实现 Flyer 和 Runner 接口
class Bird implements Flyer, Runner {
// 实现接口方法
@Override
public void fly() {
System.out.println("鸟儿正在飞翔");
}
@Override
public void run() {
System.out.println("鸟儿正在跑步 (可能是特指鸟类的一种特殊运动)");
}
}
```
接下来,我们可以创建一个测试类 `TestBird` 来检验 `Bird` 是否具备这两个接口的功能:
```java
// 测试类 TestBird
public class TestBird {
public static void main(String[] args) {
Bird bird = new Bird(); // 创建鸟的实例
// 调用 Flyer 接口的方法
bird.fly();
// 调用 Runner 接口的方法
bird.run();
// 打印结果
System.out.println("鸟的飞行和跑步能力测试完成");
}
}
```
运行这个测试类,你会看到鸟儿如何模拟飞翔和跑步的行为。这体现了多态性,因为虽然 `bird` 变量声明为 `Bird` 类型,但它可以根据实际实现的接口调用相应的行为。
阅读全文
相关推荐
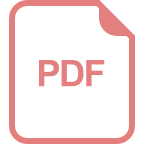
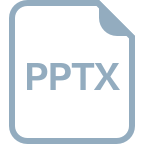
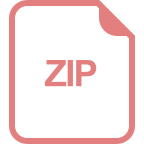
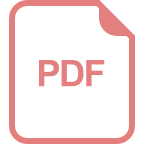
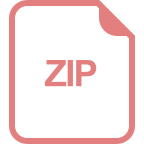
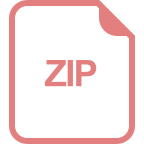
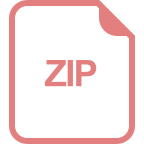
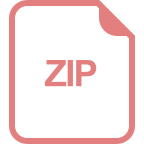
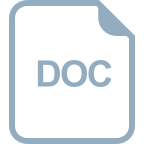






