springboot调用第三方接口工具
时间: 2023-09-13 18:13:42 浏览: 51
Spring Boot 调用第三方接口可以使用 Java 提供的 HttpURLConnection 或者第三方库如 HttpClient 和 OkHttp 等。
下面以使用 HttpClient 为例,介绍如何在 Spring Boot 中调用第三方接口。
1. 添加依赖
在项目的 pom.xml 文件中添加 HttpClient 的依赖:
```
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
```
2. 编写接口调用方法
在 Spring Boot 的服务类中,编写调用第三方接口的方法。下面是一个示例代码:
```
@Service
public class ApiService {
private CloseableHttpClient httpClient;
@PostConstruct
public void init() {
httpClient = HttpClients.createDefault();
}
public String getDataFromApi(String apiURL) throws Exception {
HttpGet request = new HttpGet(apiURL);
try (CloseableHttpResponse response = httpClient.execute(request)) {
HttpEntity entity = response.getEntity();
if (entity != null) {
return EntityUtils.toString(entity);
} else {
return null;
}
}
}
@PreDestroy
public void close() throws IOException {
httpClient.close();
}
}
```
在上面的代码中,我们使用了 HttpClient 的 `HttpGet` 请求对象,通过 `httpClient.execute(request)` 方法获取响应结果。
3. 在控制器中调用接口
在 Spring Boot 的控制器类中,注入 ApiService 对象,然后调用其中的方法即可。下面是一个示例代码:
```
@RestController
public class ApiController {
@Autowired
private ApiService apiService;
@GetMapping("/getDataFromApi")
public String getDataFromApi() throws Exception {
String apiURL = "https://api.example.com/data";
return apiService.getDataFromApi(apiURL);
}
}
```
在上面的代码中,我们使用了 `@GetMapping` 注解来定义 GET 请求,然后调用了 ApiService 中的 `getDataFromApi` 方法,将返回结果作为响应返回给客户端。
注意:在实际使用中,需要根据第三方接口的具体要求,设置请求头、请求参数等信息。
相关推荐
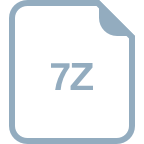
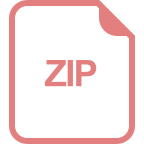














