"该资源主要介绍了如何在SpringBoot环境下使用RestTemplate进行第三方接口访问,包括POST方法传递JSON字符串、GET方法通过路径传参、POST方法传递form表单以及GET方法无参的情况。" 在Java开发中,SpringBoot是一个非常流行的微服务框架,它简化了配置并提供了快速开发新应用的能力。在SpringBoot中,我们可以利用RestTemplate工具类来方便地与外部RESTful API进行交互。以下将详细解释如何实现上述功能。 1. 添加依赖 在SpringBoot项目中,你需要确保已经添加了`spring-web`依赖,因为RestTemplate是包含在这个模块中的。通常,如果你使用的是SpringBoot初始器创建的项目,这个依赖应该已经包含在默认的`web`起步依赖中。如果不在,你可以在`pom.xml`或`build.gradle`文件中添加相应的依赖。 ```xml <!-- Maven --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> // Gradle dependencies { implementation 'org.springframework.boot:spring-boot-starter-web' } ``` 2. POST方法传递JSON字符串 使用RestTemplate发送POST请求并携带JSON数据时,可以这样做: ```java @Test public void test1() throws Exception { RestTemplate restTemplate = new RestTemplate(); String url = "https://shenmazong.com/test/sendEmail"; CommParamVo commParamVo = new CommParamVo(); commParamVo.setUserId(1001); commParamVo.setMessage("hello"); HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_JSON); HttpEntity<CommParamVo> entity = new HttpEntity<>(commParamVo, headers); ResponseEntity<String> response = restTemplate.postForEntity(url, entity, String.class); // 处理响应结果 } ``` 在这个例子中,我们创建了一个`HttpHeaders`对象,并设置其`Content-Type`为`application/json`,然后构建一个`HttpEntity`,将`CommParamVo`对象作为请求体。最后,使用`RestTemplate`的`postForEntity`方法发送请求并获取响应。 3. GET方法,通过路径传参 对于GET请求,参数通常通过URL路径或者查询参数传递。如果需要在路径中包含参数,可以这样处理: ```java @Test public void test2() { RestTemplate restTemplate = new RestTemplate(); String url = "https://shenmazong.com/test/email/{userId}"; String userId = "1001"; ResponseEntity<String> response = restTemplate.getForEntity(url.replace("{userId}", userId), String.class); // 处理响应结果 } ``` 这里,我们使用`getForEntity`方法并替换URL模板中的`{userId}`。 4. POST方法传递form表单 如果接口需要接收form表单数据,可以使用`LinkedMultiValueMap`来构造表单参数: ```java @Test public void test3() { RestTemplate restTemplate = new RestTemplate(); String url = "https://shenmazong.com/test/form"; Map<String, Object> params = new HashMap<>(); params.put("userId", 1001); params.put("message", "hello"); HttpHeaders headers = new HttpHeaders(); headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED); HttpEntity<MultiValueMap<String, Object>> entity = new HttpEntity<>(new LinkedMultiValueMap<>(params), headers); ResponseEntity<String> response = restTemplate.postForEntity(url, entity, String.class); // 处理响应结果 } ``` 5. GET方法无参 当GET请求不需要参数时,直接调用`getForEntity`即可: ```java @Test public void test4() { RestTemplate restTemplate = new RestTemplate(); String url = "https://shenmazong.com/test/noParams"; ResponseEntity<String> response = restTemplate.getForEntity(url, String.class); // 处理响应结果 } ``` 以上代码示例展示了如何在SpringBoot中使用RestTemplate与第三方接口进行交互,涵盖了常见的HTTP请求方法和数据传递方式。在实际开发中,可能还需要处理异常、超时、重试等高级用法,确保接口调用的稳定性和可靠性。
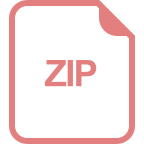


















- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 解决本地连接丢失无法上网的问题
- BIOS报警声音解析:故障原因与解决方法
- 广义均值移动跟踪算法在视频目标跟踪中的应用研究
- C++Builder快捷键大全:高效编程的秘密武器
- 网页制作入门:常用代码详解
- TX2440A开发板网络远程监控系统移植教程:易搭建与通用解决方案
- WebLogic10虚拟内存配置详解与优化技巧
- C#网络编程深度解析:Socket基础与应用
- 掌握Struts1:Java MVC轻量级框架详解
- 20个必备CSS代码段提升Web开发效率
- CSS样式大全:字体、文本、列表样式详解
- Proteus元件库大全:从基础到高级组件
- 74HC08芯片:高速CMOS四输入与门详细资料
- C#获取当前路径的多种方法详解
- 修复MySQL乱码问题:设置字符集为GB2312
- C语言的诞生与演进:从汇编到系统编程的革命

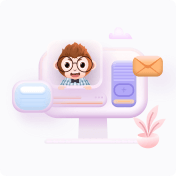
