@RequestMapping( value = {"/{user_name}/update"}, method = {RequestMethod.GET} ) public void update(@PathVariable String user_name, Model model, HttpServletRequest request, HttpServletResponse response) throws Exception { UserInfo userInfo = this.userInfoService.getUserInfo(user_name); response.setContentType("text/json;charset=UTF-8"); PrintWriter out = response.getWriter(); JSONObject jsonUserInfo = userInfo.getJsonObject(); out.println(jsonUserInfo.toString()); out.flush(); out.close(); }
时间: 2023-06-25 17:08:04 浏览: 150
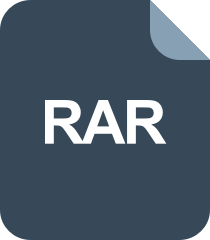
SpringMVC-2 使用@RequestMapping映射请求
这是一个使用Spring MVC框架的Java代码段,用于处理GET请求并返回用户信息的JSON对象。具体来说,它使用了@RequestMapping注释将URL映射到处理程序方法上,@PathVariable注释将URI模板变量映射到方法参数上,Model对象用于向视图层传递数据,HttpServletRequest和HttpServletResponse对象用于处理HTTP请求和响应,UserInfoService对象用于获取用户信息,JSONObject对象用于将用户信息转换为JSON格式,PrintWriter对象用于将JSON字符串写入HTTP响应中。
阅读全文
相关推荐
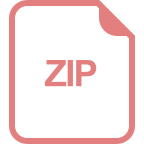
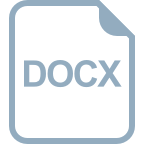















