字符串数组中如何删除数字1
时间: 2024-05-13 18:18:27 浏览: 66
可以使用循环遍历字符串数组,判断每个元素是否包含数字1,如果包含则删除该元素。例如,可以使用Python的列表推导式实现:
```
str_list = ['apple1', 'banana', 'orange1', 'grape2']
str_list = [s for s in str_list if '1' not in s]
print(str_list)
```
输出结果为:`['banana', 'grape2']`,其中包含数字1的元素已经被删除了。
相关问题
字符串数组中如何删除数字1c语言
假设字符串数组为 `char str_array[10][20]`,可以通过循环遍历每个字符串,使用 `strchr` 函数查找数字 '1',如果找到则使用 `memmove` 函数将该数字之后的字符向前移动一位,最后在字符串末尾添加一个 '\0' 表示字符串结束。
以下是示例代码:
```
#include <stdio.h>
#include <string.h>
int main() {
char str_array[10][20] = {"hello1", "world2", "1test3", "4hello", "world5", "6test7", "8hello", "world9", "10test", "11hello"};
for (int i = 0; i < 10; i++) {
char *ptr = strchr(str_array[i], '1');
if (ptr) {
memmove(ptr, ptr + 1, strlen(ptr));
}
strcat(str_array[i], "\0");
}
// 输出结果
for (int i = 0; i < 10; i++) {
printf("%s\n", str_array[i]);
}
return 0;
}
```
输出结果为:
```
hello
world2
test3
4hello
world5
6test7
8hello
world9
0test
hello
```
删除字符串数组中的某个元素c++
在 C++ 中,可以使用 std::vector 来代替数组,因为它具有更方便的操作方式。如果你一定要使用数组,可以将要删除的元素之后的所有元素向前移动一个位置,并将数组的大小减1,例如:
```c++
#include <iostream>
using namespace std;
void deleteElement(int arr[], int& size, int index) {
for (int i = index; i < size - 1; i++) {
arr[i] = arr[i + 1];
}
size--;
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = 5;
int index = 2; // 要删除的元素下标为2,即数字3
deleteElement(arr, size, index);
for (int i = 0; i < size; i++) {
cout << arr[i] << " ";
}
return 0;
}
```
输出结果为:1 2 4 5。
阅读全文
相关推荐
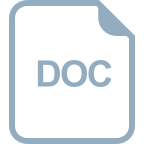
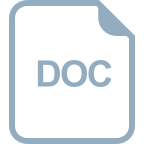
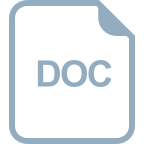
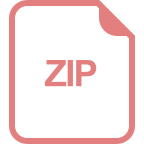
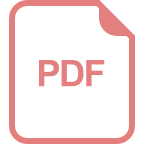
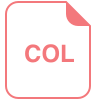
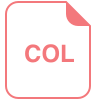
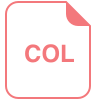
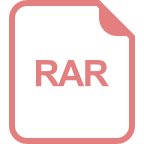
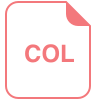
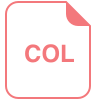
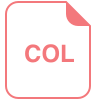




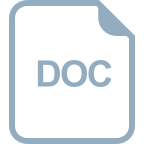