字符串数组操作全攻略:增删改查,掌握基本操作,提升开发效率
发布时间: 2024-07-09 14:40:21 阅读量: 87 订阅数: 35 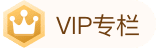
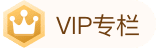
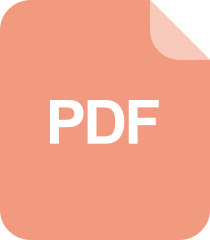
字符串数组反转全攻略:技巧、代码实现与应用场景
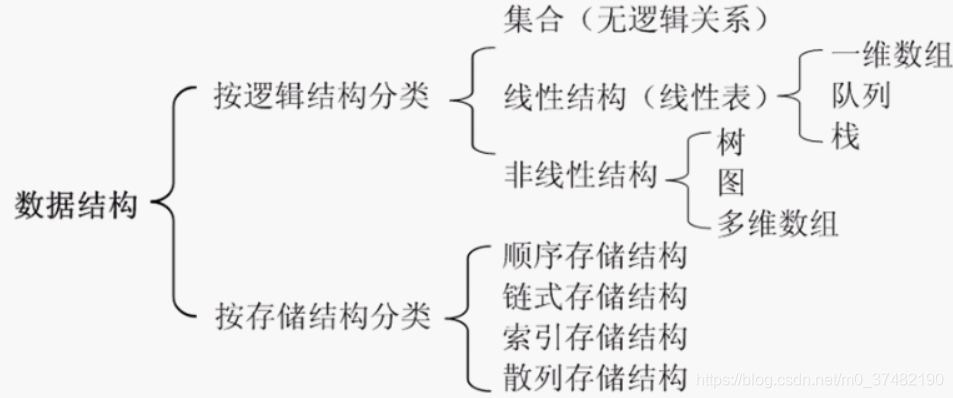
# 1. 字符串数组的基本概念和操作**
字符串数组是 Bash 脚本中用于存储字符串集合的数据结构。它本质上是一个关联数组,其中键是数字索引,而值是字符串。
**数组定义和初始化**
```bash
# 定义一个空数组
declare -a my_array
# 初始化数组元素
my_array[0]="Element 1"
my_array[1]="Element 2"
my_array[2]="Element 3"
```
**数组访问**
```bash
# 访问数组元素
echo "${my_array[1]}" # 输出:Element 2
```
# 2. 字符串数组的增删改查
字符串数组的增删改查是数组操作中最为基础和常用的操作,本章节将详细介绍如何对字符串数组进行添加、删除、修改和查找元素。
### 2.1 添加元素
添加元素是向字符串数组中插入新元素的操作,有两种常用的方法:
#### 2.1.1 使用+=运算符
+=运算符可以将一个或多个元素追加到数组的末尾。语法如下:
```
数组名+=元素1 元素2 ...
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob")
# 使用+=运算符添加元素
names+=("Alice" "Tom")
# 打印数组
echo "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含三个元素:"John"、"Mary"和"Bob"。
* 然后使用+=运算符将两个新元素:"Alice"和"Tom"追加到数组的末尾。
* 最后打印数组,输出结果为:"John Mary Bob Alice Tom"。
#### 2.1.2 使用数组赋值
数组赋值也可以用于向数组中添加元素,语法如下:
```
数组名[索引]=元素
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob")
# 使用数组赋值添加元素
names[3]="Alice"
names[4]="Tom"
# 打印数组
echo "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含三个元素:"John"、"Mary"和"Bob"。
* 然后使用数组赋值将两个新元素:"Alice"和"Tom"分别添加到数组的第4个和第5个索引位置。
* 最后打印数组,输出结果为:"John Mary Bob Alice Tom"。
### 2.2 删除元素
删除元素是从字符串数组中移除指定元素的操作,有两种常用的方法:
#### 2.2.1 使用unset命令
unset命令可以删除数组中的指定元素,语法如下:
```
unset 数组名[索引]
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob" "Alice" "Tom")
# 使用unset命令删除元素
unset names[2]
# 打印数组
echo "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含五个元素:"John"、"Mary"、"Bob"、"Alice"和"Tom"。
* 然后使用unset命令删除数组中索引为2的元素,即"Bob"。
* 最后打印数组,输出结果为:"John Mary Alice Tom"。
#### 2.2.2 使用数组切片
数组切片也可以用于删除数组中的元素,语法如下:
```
数组名=( ${数组名[@]:起始索引:结束索引} )
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob" "Alice" "Tom")
# 使用数组切片删除元素
names=( ${names[@]:1:3} )
# 打印数组
echo "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含五个元素:"John"、"Mary"、"Bob"、"Alice"和"Tom"。
* 然后使用数组切片删除数组中索引为1到3的元素,即"Mary"、"Bob"和"Alice"。
* 最后打印数组,输出结果为:"John Tom"。
### 2.3 修改元素
修改元素是从字符串数组中更新指定元素的操作,有两种常用的方法:
#### 2.3.1 使用下标赋值
下标赋值可以修改数组中指定索引的元素,语法如下:
```
数组名[索引]=元素
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob" "Alice" "Tom")
# 使用下标赋值修改元素
names[2]="David"
# 打印数组
echo "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含五个元素:"John"、"Mary"、"Bob"、"Alice"和"Tom"。
* 然后使用下标赋值将数组中索引为2的元素,即"Bob"修改为"David"。
* 最后打印数组,输出结果为:"John Mary David Alice Tom"。
#### 2.3.2 使用数组切片赋值
数组切片赋值也可以用于修改数组中的元素,语法如下:
```
数组名=( ${数组名[@]:起始索引:结束索引=元素} )
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob" "Alice" "Tom")
# 使用数组切片赋值修改元素
names=( ${names[@]:2:2=David Alice} )
# 打印数组
echo "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含五个元素:"John"、"Mary"、"Bob"、"Alice"和"Tom"。
* 然后使用数组切片赋值将数组中索引为2到3的元素,即"Bob"和"Alice"修改为"David"和"Alice"。
* 最后打印数组,输出结果为:"John Mary David Alice"。
### 2.4 查找元素
查找元素是从字符串数组中搜索指定元素的操作,有两种常用的方法:
#### 2.4.1 使用in运算符
in运算符可以判断一个元素是否在数组中,语法如下:
```
if 元素 in 数组名; then
# 元素存在
else
# 元素不存在
fi
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob" "Alice" "Tom")
# 使用in运算符查找元素
if "Bob" in "${names[@]}"; then
echo "Bob exists in the array"
else
echo "Bob does not exist in the array"
fi
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含五个元素:"John"、"Mary"、"Bob"、"Alice"和"Tom"。
* 然后使用in运算符判断元素"Bob"是否在数组中。
* 输出结果为:"Bob exists in the array"。
#### 2.4.2 使用grep命令
grep命令也可以用于从字符串数组中查找元素,语法如下:
```
grep 元素 数组名
```
**代码块:**
```bash
#!/bin/bash
# 定义一个字符串数组
declare -a names=("John" "Mary" "Bob" "Alice" "Tom")
# 使用grep命令查找元素
grep "Bob" "${names[@]}"
```
**逻辑分析:**
* 首先定义了一个字符串数组`names`,包含五个元素:"John"、"Mary"、"Bob"、"Alice"和"Tom"。
* 然后使用grep命令查找元素"Bob"。
* 输出结果为:"Bob"。
# 3. 字符串数组的遍历和排序
### 3.1 遍历数组
遍历数组是指逐个访问数组中的每个元素。在 Bash 中,有两种常用的遍历数组的方法:
#### 3.1.1 使用 for 循环
```bash
# 声明一个字符串数组
declare -a fruits=("apple" "banana" "cherry" "dog")
# 使用 for 循环遍历数组
for fruit in "${fruits[@]}"; do
echo "Fruit: $fruit"
done
```
**代码逻辑解读:**
- `declare -a fruits=("apple" "banana" "cherry" "dog")`:声明一个名为 `fruits` 的字符串数组,并初始化四个元素。
- `for fruit in "${fruits[@]}"; do`:使用 `for` 循环遍历数组 `fruits` 中的每个元素,并将其分配给变量 `fruit`。
- `echo "Fruit: $fruit"`:打印变量 `fruit` 的值,显示数组中的每个元素。
#### 3.1.2 使用 while 循环
```bash
# 声明一个字符串数组
declare -a fruits=("apple" "banana" "cherry" "dog")
# 使用 while 循环遍历数组
index=0
while [ $index -lt ${#fruits[@]} ]; do
echo "Fruit: ${fruits[$index]}"
index=$((index + 1))
done
```
**代码逻辑解读:**
- `declare -a fruits=("apple" "banana" "cherry" "dog")`:声明一个名为 `fruits` 的字符串数组,并初始化四个元素。
- `index=0`:初始化一个变量 `index`,用于跟踪数组中的当前索引。
- `while [ $index -lt ${#fruits[@]} ]; do`:使用 `while` 循环遍历数组 `fruits` 中的每个元素,直到 `index` 达到数组长度(`#fruits[@]`)。
- `echo "Fruit: ${fruits[$index]}"`:打印数组 `fruits` 中当前索引 `index` 处的元素。
- `index=$((index + 1))`:将 `index` 递增 1,以遍历数组中的下一个元素。
### 3.2 排序数组
排序数组是指将数组中的元素按照特定顺序排列。在 Bash 中,有两种常用的排序数组的方法:
#### 3.2.1 使用 sort 命令
```bash
# 声明一个字符串数组
declare -a fruits=("apple" "banana" "cherry" "dog")
# 使用 sort 命令对数组进行排序
sorted_fruits=($(sort <<<"${fruits[@]}"))
# 打印排序后的数组
echo "Sorted fruits: ${sorted_fruits[@]}"
```
**代码逻辑解读:**
- `declare -a fruits=("apple" "banana" "cherry" "dog")`:声明一个名为 `fruits` 的字符串数组,并初始化四个元素。
- `sorted_fruits=($(sort <<<"${fruits[@]}"))`:使用 `sort` 命令对数组 `fruits` 进行排序,并将排序后的结果存储在数组 `sorted_fruits` 中。
- `echo "Sorted fruits: ${sorted_fruits[@]}"`:打印排序后的数组 `sorted_fruits`。
#### 3.2.2 使用自定义比较函数
```bash
# 声明一个字符串数组
declare -a fruits=("apple" "banana" "cherry" "dog")
# 定义一个自定义比较函数
compare() {
[[ "$1" > "$2" ]]
}
# 使用 sort 命令对数组进行排序,并指定自定义比较函数
sorted_fruits=($(sort -t ' ' -k 1,1 <<<"${fruits[@]}" -c compare))
# 打印排序后的数组
echo "Sorted fruits: ${sorted_fruits[@]}"
```
**代码逻辑解读:**
- `declare -a fruits=("apple" "banana" "cherry" "dog")`:声明一个名为 `fruits` 的字符串数组,并初始化四个元素。
- `compare()`:定义一个自定义比较函数,用于比较两个字符串。如果第一个字符串大于第二个字符串,则返回真(`true`)。
- `sorted_fruits=($(sort -t ' ' -k 1,1 <<<"${fruits[@]}" -c compare))`:使用 `sort` 命令对数组 `fruits` 进行排序,指定以下选项:
- `-t ' '`:使用空格作为分隔符。
- `-k 1,1`:根据第一个字段(即整个字符串)进行排序。
- `-c compare`:使用自定义比较函数 `compare`。
- `echo "Sorted fruits: ${sorted_fruits[@]}"`:打印排序后的数组 `sorted_fruits`。
# 4. 字符串数组的实用技巧
### 4.1 数组长度和元素数量
在某些情况下,我们需要知道字符串数组的长度或元素数量。Bash 提供了两种方法来获取这些信息:
- **使用 # 数组名**:此方法返回数组中元素的数量。例如:
```bash
array=(1 2 3 4 5)
echo ${#array} # 输出:5
```
- **使用 ${#数组名[@]}**:此方法返回数组中所有元素的总长度(包括每个元素的字符数)。例如:
```bash
array=(hello world 123)
echo ${#array[@]} # 输出:12
```
### 4.2 数组转字符串
将字符串数组转换为单个字符串在许多情况下很有用,例如在需要将数组内容传递给其他命令时。Bash 提供了两种方法来实现此目的:
- **使用 IFS 变量**:IFS(内部字段分隔符)变量指定用于分隔数组元素的字符。默认情况下,IFS 为空格。我们可以通过修改 IFS 来将数组元素连接成一个字符串。例如:
```bash
array=(1 2 3 4 5)
IFS=,
echo ${array[*]} # 输出:1,2,3,4,5
```
- **使用 printf 命令**:printf 命令可以格式化输出。我们可以使用 %s 格式说明符来打印数组元素,并使用分隔符将其连接起来。例如:
```bash
array=(1 2 3 4 5)
printf "%s," ${array[*]} # 输出:1,2,3,4,5,
```
### 4.3 字符串转数组
将字符串转换为字符串数组也很有用,例如在需要从文件中读取数据或从用户输入中解析数据时。Bash 提供了两种方法来实现此目的:
- **使用 read 命令**:read 命令可以从标准输入读取一行或多行文本。我们可以使用 -a 选项将输入存储到一个数组中。例如:
```bash
read -a array
echo ${array[0]} # 输出:第一行输入
echo ${array[1]} # 输出:第二行输入
```
- **使用 IFS 变量**:与数组转字符串类似,我们可以使用 IFS 变量来将字符串拆分为数组元素。例如:
```bash
string="hello world 123"
IFS=' '
read -a array <<< "$string"
echo ${array[0]} # 输出:hello
echo ${array[1]} # 输出:world
echo ${array[2]} # 输出:123
```
# 5.1 数组的嵌套和多维数组
### 5.1.1 嵌套数组的定义和访问
嵌套数组是指数组中包含另一个或多个数组。嵌套数组的定义方式如下:
```bash
array_name=(element1 element2 (element3 element4))
```
其中,`element3` 和 `element4` 构成了一个嵌套数组。
要访问嵌套数组中的元素,可以使用以下语法:
```bash
array_name[index1][index2]
```
其中,`index1` 是外层数组的索引,`index2` 是嵌套数组的索引。
**示例:**
```bash
nested_array=(1 2 (3 4))
echo ${nested_array[0]} # 输出:1
echo ${nested_array[1]} # 输出:2
echo ${nested_array[2][0]} # 输出:3
echo ${nested_array[2][1]} # 输出:4
```
### 5.1.2 多维数组的定义和访问
多维数组是指嵌套多个数组的数组。多维数组的定义方式如下:
```bash
array_name=(element1 element2 (element3 element4 (element5 element6)))
```
其中,`element3`、`element4`、`element5` 和 `element6` 构成了一个多维数组。
要访问多维数组中的元素,可以使用以下语法:
```bash
array_name[index1][index2][index3]
```
其中,`index1` 是最外层数组的索引,`index2` 是中间层数组的索引,`index3` 是最内层数组的索引。
**示例:**
```bash
multi_array=(1 2 (3 4 (5 6)))
echo ${multi_array[0]} # 输出:1
echo ${multi_array[1]} # 输出:2
echo ${multi_array[2][0]} # 输出:3
echo ${multi_array[2][1][0]} # 输出:5
echo ${multi_array[2][1][1]} # 输出:6
```
0
0
相关推荐






