装饰器模式-实现电商的优惠活动价格计算
时间: 2023-07-07 07:06:11 浏览: 45
装饰器模式是一种结构型设计模式,它可以动态地给对象添加额外的职责,同时又不需要修改原有的代码。在电商领域中,我们可以使用装饰器模式来实现优惠活动价格计算。
首先,我们需要定义一个抽象组件类,代表商品或服务:
```python
from abc import ABC, abstractmethod
class Component(ABC):
@abstractmethod
def get_price(self):
pass
```
然后,我们定义具体的商品类:
```python
class Product(Component):
def __init__(self, name, price):
self.name = name
self.price = price
def get_price(self):
return self.price
```
接下来,我们定义装饰器类:
```python
class Decorator(Component):
def __init__(self, component):
self.component = component
def get_price(self):
return self.component.get_price()
```
其中,`Decorator` 类继承自 `Component` 类,它包含一个对抽象组件的引用,代表要装饰的组件。
然后,我们定义具体的装饰器类,用来实现各种优惠活动:
```python
class Discount(Decorator):
def __init__(self, component, discount_rate):
super().__init__(component)
self.discount_rate = discount_rate
def get_price(self):
return self.component.get_price() * self.discount_rate
```
`Discount` 类继承自 `Decorator` 类,它包含一个对抽象组件的引用,同时还有一个折扣率属性 `discount_rate`。`get_price` 方法将原始价格与折扣率相乘,得到折扣后的价格。
我们还可以定义其他的装饰器类,如满减、满赠等等。
最后,我们可以使用这些装饰器类来计算商品的最终价格:
```python
product = Product('iPhone', 10000)
discounted_product = Discount(product, 0.8) # 打八折
print(discounted_product.get_price()) # 输出 8000
```
这样,我们就通过装饰器模式实现了电商的优惠活动价格计算。
相关推荐
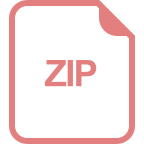
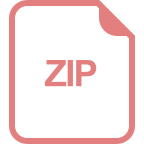
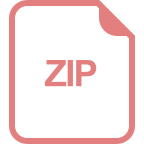














