Create a test class with three static methods shown below at minimum. public class Test{ public static void start(){ - Create an empty list of shapes and save the reference. - Pass the reference to create method that fills the list. - Pass the reference to display method that prints the list. //The following can be added here or into an additional method. - Remove a shape. - Display the list again. - Check the size of the list. - … } public static returnTypeOrVoid create(a reference to a list) { - Create shape objects using data from an input file. - Note: A shape can be a rectangle, a circle or a triangle. - Add the objects into the list. } public static returnTypeOrVoid display(a reference to a list) { - Display the objects of the list. … } }
时间: 2023-11-22 09:53:36 浏览: 59
Here is an example implementation of the Test class with the three static methods:
```
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import java.io.File;
import java.io.FileNotFoundException;
public class Test {
public static void start() {
// Create an empty list of shapes
List<Shape> shapes = new ArrayList<>();
// Fill the list with shapes from input file
create(shapes);
// Print the list of shapes
System.out.println("List of Shapes:");
display(shapes);
// Remove a shape from the list
if (!shapes.isEmpty()) {
Shape removedShape = shapes.remove(0);
System.out.println("Removed Shape: " + removedShape);
}
// Print the updated list of shapes
System.out.println("Updated List of Shapes:");
display(shapes);
// Check the size of the list
System.out.println("Size of List of Shapes: " + shapes.size());
}
public static void create(List<Shape> shapes) {
// Read input file and create shape objects
try {
Scanner scanner = new Scanner(new File("shapes.txt"));
while (scanner.hasNextLine()) {
String[] tokens = scanner.nextLine().split(",");
String shapeType = tokens[0].trim();
double width = Double.parseDouble(tokens[1].trim());
double height = Double.parseDouble(tokens[2].trim());
switch (shapeType) {
case "rectangle":
shapes.add(new Rectangle(width, height));
break;
case "circle":
shapes.add(new Circle(width / 2));
break;
case "triangle":
shapes.add(new Triangle(width, height));
break;
default:
System.out.println("Invalid Shape Type: " + shapeType);
break;
}
}
scanner.close();
} catch (FileNotFoundException e) {
System.out.println("File not found: shapes.txt");
}
}
public static void display(List<Shape> shapes) {
// Print each shape in the list
for (Shape shape : shapes) {
System.out.println(shape);
}
}
}
```
This Test class reads a file called "shapes.txt" that contains information about each shape, including its type, width, and height. It creates the appropriate shape objects based on the type, adds them to the list, and then prints the list. It also demonstrates removing a shape from the list, printing the updated list, and checking the size of the list. You can modify the implementation according to your requirements.
相关推荐
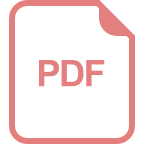
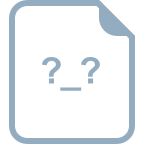
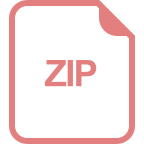














