public static File reset(File htmlFile,String htmlPath,String reportId){ File newHtmlFile = null; try { BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(htmlFile), "UTF-8")); StringBuilder stringBuilder = new StringBuilder(); String line = null; while ((line = reader.readLine()) != null) { stringBuilder.append(line); stringBuilder.append(System.lineSeparator()); } reader.close(); String str = stringBuilder.toString(); System.out.println(str); // 解析HTML文件 Document doc = Jsoup.parse(str); /*// 找到目标div标签 Element div = doc.getElementById("null_background"); // 修改style属性中的背景样式 div.attr("style", div.attr("style").replace("background:", "")); // 生成新的File对象*/ Path newFilePath = Paths.get(htmlPath+"/reseHtml/"+reportId + ".html"); Files.write(newFilePath, doc.outerHtml().getBytes()); newHtmlFile = new File(newFilePath.toString()); return newHtmlFile; }catch (Exception o){ o.printStackTrace(); newHtmlFile = htmlFile; } return newHtmlFile; }生成的html乱码了怎么处理
时间: 2024-02-14 16:24:19 浏览: 143
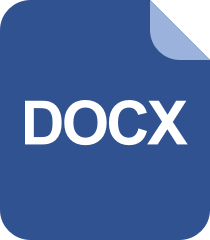
文档格式转换 html to word
出现乱码可能是因为编码不一致导致的。可以尝试以下几种解决方法:
1. 在读取文件时指定正确的编码格式,例如读取 UTF-8 编码的文件应使用 `new InputStreamReader(new FileInputStream(htmlFile), "UTF-8")`。
2. 在生成新的 HTML 文件时,指定正确的编码格式,例如使用 `Files.write(newFilePath, doc.outerHtml().getBytes("UTF-8"))`。
3. 在 HTML 文件的头部添加编码声明,例如 `<meta charset="UTF-8">`,这样浏览器就能正确地解析文件中的非 ASCII 字符。
如果以上方法还是无法解决问题,可能是因为文件本身出现了损坏或错误,需要检查原始文件是否正确无误。
阅读全文
相关推荐
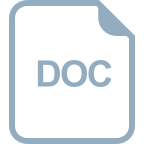
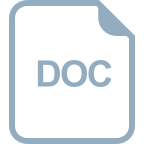
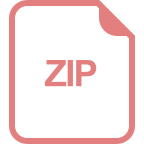
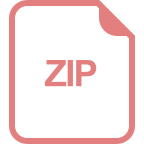
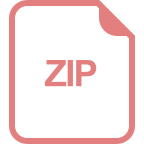
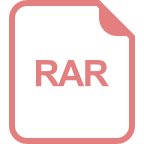
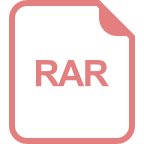
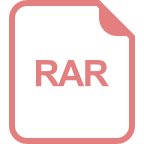
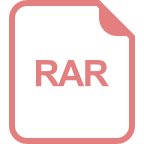
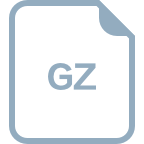
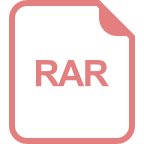






