用python写一个折线代码+图形+html
时间: 2023-06-12 21:05:48 浏览: 311
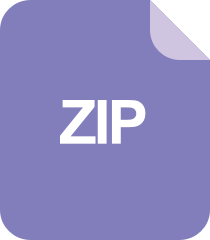
python实战:画组合图形.zip
可以使用Python的Matplotlib库来生成折线图,并使用HTML将其展示在网页上。以下是一个简单的示例:
```python
import matplotlib.pyplot as plt
# 创建数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 设置图形属性
plt.title("折线图")
plt.xlabel("x轴")
plt.ylabel("y轴")
# 保存图形
plt.savefig("line_chart.png")
# 将图形以HTML格式展示在网页上
html = '<img src="line_chart.png">'
with open("line_chart.html", "w") as f:
f.write(html)
```
运行上述代码后,将生成一个名为`line_chart.png`的图片文件和一个名为`line_chart.html`的网页文件。在浏览器中打开`line_chart.html`文件,即可看到生成的折线图。
阅读全文
相关推荐
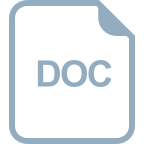
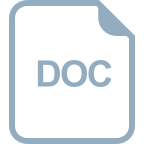
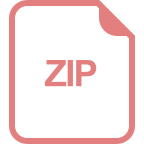
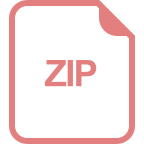
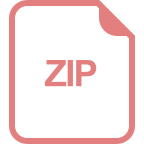
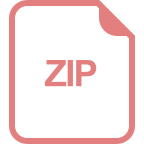
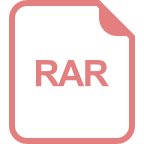
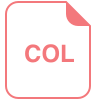






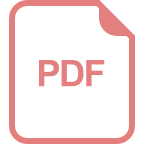
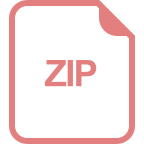
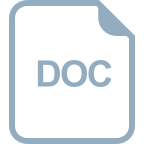
