用C语言写一个链表的代码,输入一个整数,打印链表。如果链表中已经有了输入的整数,则将输入的数乘以2在打印链表
时间: 2024-03-13 08:25:58 浏览: 59
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建链表节点
struct Node* createNode(int data) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->data = data;
node->next = NULL;
return node;
}
// 插入节点到链表尾部
void insertAtEnd(struct Node** head, int data) {
struct Node* node = createNode(data);
if (*head == NULL) {
*head = node;
} else {
struct Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = node;
}
}
// 打印链表
void printList(struct Node* head) {
if (head == NULL) {
printf("链表为空\n");
} else {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
}
// 查找链表中是否存在某个元素
int searchElement(struct Node* head, int data) {
struct Node* current = head;
while (current != NULL) {
if (current->data == data) {
return 1;
}
current = current->next;
}
return 0;
}
// 修改链表元素
void modifyElement(struct Node* head, int data) {
struct Node* current = head;
while (current != NULL) {
if (current->data == data) {
current->data *= 2;
}
current = current->next;
}
}
int main() {
struct Node* head = NULL;
int n;
printf("请输入整数:");
scanf("%d", &n);
if (searchElement(head, n)) {
n *= 2;
}
insertAtEnd(&head, n);
printf("当前链表:");
printList(head);
return 0;
}
阅读全文
相关推荐
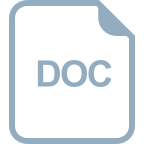
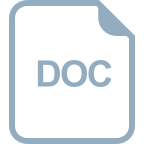
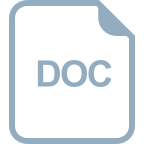















