用java语句判断ChengYu类的列表chengYuList中是否有字符串chengyu
时间: 2024-02-29 11:52:37 浏览: 60
可以使用Java中的for循环遍历ChengYu类的列表chengYuList,然后判断每个ChengYu类实例的chengyu属性是否等于字符串chengyu,如果等于,则说明chengYuList中存在该字符串。可以使用如下代码实现:
```
boolean isExist = false;
for (ChengYu chengYu : chengYuList) {
if (chengYu.getChengyu().equals(chengyu)) {
isExist = true;
break;
}
}
if (isExist) {
System.out.println("chengYuList中存在字符串" + chengyu);
} else {
System.out.println("chengYuList中不存在字符串" + chengyu);
}
```
其中,ChengYu类需要提供获取chengyu属性的方法getChengyu(),并且使用equals()方法判断字符串是否相等。最后使用一个布尔变量isExist来记录是否存在该字符串,如果存在,则将isExist设置为true,同时跳出循环。最后根据isExist的值输出结果。
相关问题
用java语句判断ChengYu类的列表chengYuList中是否有字符串answer,其中ChengYu类中包含方法getChengYu可以得到字符串
可以使用如下的Java语句来判断:
```java
boolean containsAnswer = false;
for (ChengYu chengYu : chengYuList) {
if (chengYu.getChengYu().equals(answer)) {
containsAnswer = true;
break;
}
}
```
其中,我们遍历了chengYuList列表中的每一个ChengYu对象,通过调用getChengYu方法获取其对应的字符串,然后与answer比较,如果相等就标记containsAnswer为true,表示列表中包含了这个字符串。如果遍历完整个列表仍然没有找到匹配的字符串,那么containsAnswer就会保持为false。
判断ChengYu类的列表chengYuList中是否有字符串chengyu
可以使用Python的in关键字来判断列表中是否存在某个元素,因此可以通过以下代码来判断ChengYu类的列表chengYuList中是否有字符串chengyu:
```
if chengyu in [item.chengyu for item in chengYuList]:
print("chengYuList中存在字符串{}".format(chengyu))
else:
print("chengYuList中不存在字符串{}".format(chengyu))
```
其中,item.chengyu表示获取ChengYu类实例的chengyu属性,最后使用列表推导式将所有的chengyu属性组成一个列表,然后判断chengyu是否在这个列表中即可。
阅读全文
相关推荐
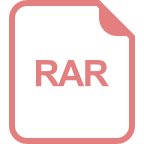
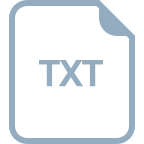













