改造Set类,重载+(并集)、(-)差集、*(交集)、<<(输出)、>>(输入)和函数调用操作符(添加一个元素)
时间: 2023-10-26 15:07:13 浏览: 48
以下是改造后的Set类的代码,包括重载的操作符和函数:
```C++
#include <iostream>
#include <set>
using namespace std;
class Set
{
private:
set<int> s;
public:
Set() {}
Set(initializer_list<int> l)
{
for (auto i : l)
{
s.insert(i);
}
}
Set operator+(const Set &other) const
{
Set result;
set_union(s.begin(), s.end(), other.s.begin(), other.s.end(), inserter(result.s, result.s.begin()));
return result;
}
Set operator-(const Set &other) const
{
Set result;
set_difference(s.begin(), s.end(), other.s.begin(), other.s.end(), inserter(result.s, result.s.begin()));
return result;
}
Set operator*(const Set &other) const
{
Set result;
set_intersection(s.begin(), s.end(), other.s.begin(), other.s.end(), inserter(result.s, result.s.begin()));
return result;
}
friend ostream &operator<<(ostream &out, const Set &set)
{
out << "{ ";
for (auto i : set.s)
{
out << i << " ";
}
out << "}";
return out;
}
friend istream &operator>>(istream &in, Set &set)
{
int num;
cout << "Enter element (-1 to stop): ";
while (true)
{
in >> num;
if (num == -1)
{
break;
}
set.s.insert(num);
cout << "Enter element (-1 to stop): ";
}
return in;
}
void operator()(int num)
{
s.insert(num);
}
};
```
使用示例:
```C++
int main()
{
Set a = {1, 2, 3, 4};
Set b = {3, 4, 5, 6};
Set c = a + b;
Set d = a - b;
Set e = a * b;
cout << "a: " << a << endl;
cout << "b: " << b << endl;
cout << "a + b: " << c << endl;
cout << "a - b: " << d << endl;
cout << "a * b: " << e << endl;
Set f;
cin >> f;
cout << "f: " << f << endl;
f(10);
cout << "f: " << f << endl;
return 0;
}
```
输出结果:
```
a: { 1 2 3 4 }
b: { 3 4 5 6 }
a + b: { 1 2 3 4 5 6 }
a - b: { 1 2 }
a * b: { 3 4 }
Enter element (-1 to stop): 7
Enter element (-1 to stop): 8
Enter element (-1 to stop): -1
f: { 7 8 }
f: { 7 8 10 }
```
相关推荐
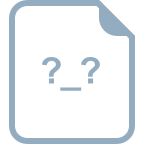
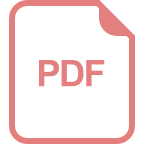
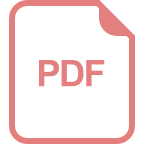
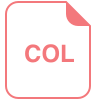
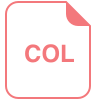












