给我一个卷积神经网络的代码
时间: 2023-11-13 21:04:26 浏览: 31
以下是一个简单的卷积神经网络的代码,用于分类MNIST手写数字图像:
```
import tensorflow as tf
# 定义输入和输出
x = tf.placeholder(tf.float32, [None, 784])
y_true = tf.placeholder(tf.float32, [None, 10])
# 将输入转换为28x28的图像
x_image = tf.reshape(x, [-1, 28, 28, 1])
# 定义第一层卷积层
conv1 = tf.layers.conv2d(inputs=x_image, filters=32, kernel_size=[5, 5], padding='same', activation=tf.nn.relu)
# 定义第一层池化层
pool1 = tf.layers.max_pooling2d(inputs=conv1, pool_size=[2, 2], strides=2)
# 定义第二层卷积层
conv2 = tf.layers.conv2d(inputs=pool1, filters=64, kernel_size=[5, 5], padding='same', activation=tf.nn.relu)
# 定义第二层池化层
pool2 = tf.layers.max_pooling2d(inputs=conv2, pool_size=[2, 2], strides=2)
# 将卷积层输出的张量转换为全连接层需要的形状
pool2_flat = tf.reshape(pool2, [-1, 7 * 7 * 64])
# 定义全连接层
dense = tf.layers.dense(inputs=pool2_flat, units=1024, activation=tf.nn.relu)
# 添加dropout,防止过拟合
dropout = tf.layers.dropout(inputs=dense, rate=0.4)
# 定义输出层
y_pred = tf.layers.dense(inputs=dropout, units=10)
# 定义损失函数
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y_true, logits=y_pred))
# 定义优化器
train_step = tf.train.AdamOptimizer(1e-4).minimize(cross_entropy)
# 定义评估模型的准确率
correct_prediction = tf.equal(tf.argmax(y_pred, 1), tf.argmax(y_true, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 初始化变量
init = tf.global_variables_initializer()
# 加载MNIST数据集
mnist = tf.contrib.learn.datasets.load_dataset("mnist")
# 将训练集和测试集分别存储为numpy数组
train_data = mnist.train.images
train_labels = mnist.train.labels.astype(int)
test_data = mnist.test.images
test_labels = mnist.test.labels.astype(int)
# 开始训练模型
with tf.Session() as sess:
sess.run(init)
for i in range(2000):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y_true: batch_ys})
if i % 100 == 0:
train_accuracy = accuracy.eval(feed_dict={x: batch_xs, y_true: batch_ys})
print("Step %d, training accuracy %g" % (i, train_accuracy))
test_accuracy = accuracy.eval(feed_dict={x: test_data, y_true: test_labels})
print("Test accuracy: %g" % test_accuracy)
```
相关推荐
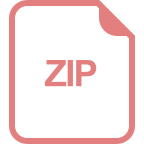














