写一个能运行的bert-bilstm-attention代码
时间: 2023-12-18 11:04:57 浏览: 92
好的,以下是一个简单的BERT-BiLSTM-Attention模型的实现代码:
```python
import torch
import torch.nn as nn
from transformers import BertModel
class BERTBiLSTMAttention(nn.Module):
def __init__(self, bert_model, hidden_size, output_size, num_layers, dropout_prob):
super(BERTBiLSTMAttention, self).__init__()
self.bert = BertModel.from_pretrained(bert_model)
self.bilstm = nn.LSTM(input_size=hidden_size, hidden_size=hidden_size, num_layers=num_layers, batch_first=True, bidirectional=True)
self.attention = nn.Linear(hidden_size*2, 1)
self.dropout = nn.Dropout(dropout_prob)
self.fc = nn.Linear(hidden_size*2, output_size)
def forward(self, input_ids, attention_mask):
bert_output = self.bert(input_ids=input_ids, attention_mask=attention_mask)
hidden_states = bert_output.last_hidden_state
lstm_output, _ = self.bilstm(hidden_states)
lstm_output = self.dropout(lstm_output)
attention_output = self.attention(lstm_output).squeeze()
attention_weights = torch.softmax(attention_output, dim=1).unsqueeze(-1)
context_vector = (lstm_output * attention_weights).sum(dim=1)
output = self.fc(context_vector)
return output
```
其中,`BertModel`来自于`transformers`库,用于加载预训练的BERT模型。在模型中,首先使用BERT对输入进行编码,然后将编码结果传入一个双向LSTM中进行序列建模,再使用一个全连接层将LSTM的输出映射到目标空间,并使用注意力机制来加强模型的表达能力。最后,使用一个全连接层将注意力机制得到的上下文向量映射到最终的输出空间。
阅读全文
相关推荐
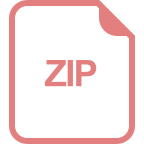
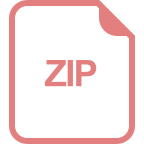
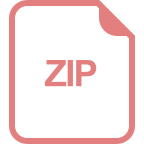
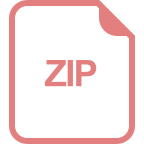
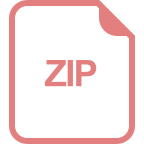
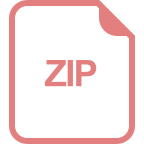
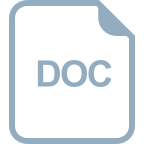
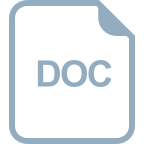
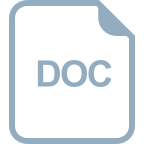
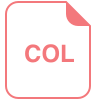
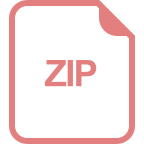
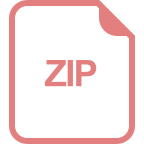
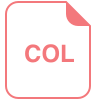
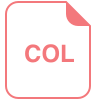
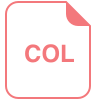
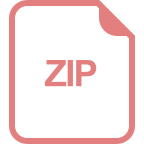