用C语言编写一段短程序代码包含列表,堆栈,队列
时间: 2023-05-28 18:07:12 浏览: 36
以下是一个简单的程序,包含列表、堆栈和队列的实现:
```c
#include <stdio.h>
// 列表
#define MAX_LIST_SIZE 10
int list[MAX_LIST_SIZE];
int list_size = 0;
void add_to_list(int value) {
if (list_size < MAX_LIST_SIZE) {
list[list_size] = value;
list_size++;
} else {
printf("Error: list is full\n");
}
}
void print_list() {
printf("List contents: [");
for (int i = 0; i < list_size; i++) {
printf("%d", list[i]);
if (i < list_size - 1) {
printf(", ");
}
}
printf("]\n");
}
// 堆栈
#define MAX_STACK_SIZE 10
int stack[MAX_STACK_SIZE];
int top = -1;
void push(int value) {
if (top < MAX_STACK_SIZE - 1) {
top++;
stack[top] = value;
} else {
printf("Error: stack overflow\n");
}
}
int pop() {
if (top >= 0) {
int value = stack[top];
top--;
return value;
} else {
printf("Error: stack underflow\n");
return -1;
}
}
void print_stack() {
printf("Stack contents: [");
for (int i = top; i >= 0; i--) {
printf("%d", stack[i]);
if (i > 0) {
printf(", ");
}
}
printf("]\n");
}
// 队列
#define MAX_QUEUE_SIZE 10
int queue[MAX_QUEUE_SIZE];
int front = 0;
int rear = -1;
int queue_size = 0;
void enqueue(int value) {
if (queue_size < MAX_QUEUE_SIZE) {
rear++;
if (rear == MAX_QUEUE_SIZE) {
rear = 0;
}
queue[rear] = value;
queue_size++;
} else {
printf("Error: queue is full\n");
}
}
int dequeue() {
if (queue_size > 0) {
int value = queue[front];
front++;
if (front == MAX_QUEUE_SIZE) {
front = 0;
}
queue_size--;
return value;
} else {
printf("Error: queue is empty\n");
return -1;
}
}
void print_queue() {
printf("Queue contents: [");
if (queue_size > 0) {
printf("%d", queue[front]);
for (int i = front + 1; i <= rear; i++) {
printf(", %d", queue[i]);
}
}
printf("]\n");
}
int main() {
add_to_list(5);
add_to_list(10);
add_to_list(15);
print_list();
push(1);
push(2);
push(3);
print_stack();
pop();
print_stack();
enqueue(1);
enqueue(2);
enqueue(3);
print_queue();
dequeue();
print_queue();
return 0;
}
```
该程序定义了三个数据结构:列表、堆栈和队列。每个数据结构都有添加、删除和打印等基本操作。程序的主函数演示了如何使用这些数据结构来存储和操作数据。注意,这只是一个简单的示例程序,实际应用中可能需要更复杂的数据结构和操作。
相关推荐
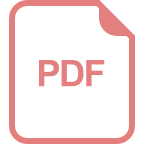














