设计一个简易的单片机十字路口交通灯,要求一共12只LED发光二极管,红绿黄各4只,红灯时间为15秒,绿灯剩余15秒时转换为黄灯,附代码
时间: 2024-04-07 14:28:49 浏览: 12
好的,这是一个简单的单片机十字路口交通灯的设计方案,并且提供基于 Keil MDK-ARM 编译器的代码实现。下面是具体的实现步骤:
1. 硬件连接
将四个东西向的LED连接在单片机的P1.0~P1.3口,将四个南北向的LED连接在单片机的P2.0~P2.3口。
2. 定义引脚
通过程序定义P1.0~P1.3口和P2.0~P2.3口为输出引脚。
```c
sbit east_west_green_light=P1^0;
sbit east_west_yellow_light=P1^1;
sbit east_west_red_light=P1^2;
sbit north_south_green_light=P2^0;
sbit north_south_yellow_light=P2^1;
sbit north_south_red_light=P2^2;
```
3. 定义变量
定义一个变量表示当前交通信号灯的状态,例如用0表示东西向为绿灯,1表示东西向为黄灯,2表示南北向为绿灯,3表示南北向为黄灯。
定义一个变量表示当前红灯的计时时间,例如用一个定时器中断每1秒减少1,当计时时间为0时,交通信号灯状态转换为下一个状态。
```c
unsigned char traffic_light_state = 0; // 交通灯状态
unsigned char red_time_left = 15; // 红灯剩余时间
```
4. 状态转换
根据交通信号灯的状态,分别控制不同的LED亮灭,例如当状态为0时,东西向为绿灯,南北向为红灯,此时将P1.0~P1.3口输出高电平,P2.0~P2.3口输出低电平。
当计时时间减少到15秒时,交通信号灯状态转换为下一个状态,即状态为1,此时将P1.0~P1.3口输出高电平,P2.0~P2.3口输出低电平,持续3秒。
当计时时间减少到18秒时,交通信号灯状态转换为下一个状态,即状态为2,此时将P2.0~P2.3口输出高电平,P1.0~P1.3口输出低电平。
当计时时间减少到33秒时,交通信号灯状态转换为下一个状态,即状态为3,此时将P2.0~P2.3口输出高电平,P1.0~P1.3口输出低电平,持续3秒。
```c
void traffic_light_control() {
switch (traffic_light_state) {
case 0: // 东西向绿灯,南北向红灯
east_west_green_light = 1;
east_west_yellow_light = 0;
east_west_red_light = 0;
north_south_green_light = 0;
north_south_yellow_light = 0;
north_south_red_light = 1;
break;
case 1: // 东西向黄灯,南北向红灯
east_west_green_light = 0;
east_west_yellow_light = 1;
east_west_red_light = 0;
north_south_green_light = 0;
north_south_yellow_light = 0;
north_south_red_light = 1;
break;
case 2: // 东西向红灯,南北向绿灯
east_west_green_light = 0;
east_west_yellow_light = 0;
east_west_red_light = 1;
north_south_green_light = 1;
north_south_yellow_light = 0;
north_south_red_light = 0;
break;
case 3: // 东西向红灯,南北向黄灯
east_west_green_light = 0;
east_west_yellow_light = 0;
east_west_red_light = 1;
north_south_green_light = 0;
north_south_yellow_light = 1;
north_south_red_light = 0;
break;
default:
break;
}
}
void timer0_interrupt() interrupt 1 {
TH0 = 0x3C;
TL0 = 0xAF;
red_time_left--;
if (red_time_left == 0) {
traffic_light_state = (traffic_light_state + 1) % 4; // 状态转换
red_time_left = 15; // 重新计时
if (traffic_light_state == 1 || traffic_light_state == 3) {
red_time_left = 3; // 黄灯时间为3秒
}
}
traffic_light_control(); // 控制交通灯
}
```
5. 循环执行
通过循环执行中断服务函数,实现交通信号灯的循环控制,即不断更新交通信号灯的状态和计时时间,控制LED的亮灭。
```c
void main() {
TMOD = 0x01; // 定时器0,16位自动重装载
TH0 = 0x3C;
TL0 = 0xAF;
ET0 = 1; // 开启定时器0中断
EA = 1; // 开启总中断
TR0 = 1; // 启动定时器0
while (1) {
}
}
```
完整代码如下所示:
```c
#include <reg52.h>
sbit east_west_green_light=P1^0;
sbit east_west_yellow_light=P1^1;
sbit east_west_red_light=P1^2;
sbit north_south_green_light=P2^0;
sbit north_south_yellow_light=P2^1;
sbit north_south_red_light=P2^2;
unsigned char traffic_light_state = 0; // 交通灯状态
unsigned char red_time_left = 15; // 红灯剩余时间
void traffic_light_control() {
switch (traffic_light_state) {
case 0: // 东西向绿灯,南北向红灯
east_west_green_light = 1;
east_west_yellow_light = 0;
east_west_red_light = 0;
north_south_green_light = 0;
north_south_yellow_light = 0;
north_south_red_light = 1;
break;
case 1: // 东西向黄灯,南北向红灯
east_west_green_light = 0;
east_west_yellow_light = 1;
east_west_red_light = 0;
north_south_green_light = 0;
north_south_yellow_light = 0;
north_south_red_light = 1;
break;
case 2: // 东西向红灯,南北向绿灯
east_west_green_light = 0;
east_west_yellow_light = 0;
east_west_red_light = 1;
north_south_green_light = 1;
north_south_yellow_light = 0;
north_south_red_light = 0;
break;
case 3: // 东西向红灯,南北向黄灯
east_west_green_light = 0;
east_west_yellow_light = 0;
east_west_red_light = 1;
north_south_green_light = 0;
north_south_yellow_light = 1;
north_south_red_light = 0;
break;
default:
break;
}
}
void timer0_interrupt() interrupt 1 {
TH0 = 0x3C;
TL0 = 0xAF;
red_time_left--;
if (red_time_left == 0) {
traffic_light_state = (traffic_light_state + 1) % 4; // 状态转换
red_time_left = 15; // 重新计时
if (traffic_light_state == 1 || traffic_light_state == 3) {
red_time_left = 3; // 黄灯时间为3秒
}
}
traffic_light_control(); // 控制交通灯
}
void main() {
TMOD = 0x01; // 定时器0,16位自动重装载
TH0 = 0x3C;
TL0 = 0xAF;
ET0 = 1; // 开启定时器0中断
EA = 1; // 开启总中断
TR0 = 1; // 启动定时器0
while (1) {
}
}
```
相关推荐
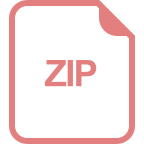
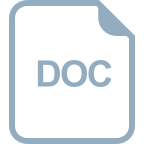
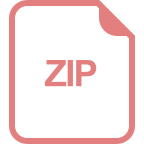
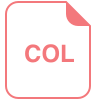














