生成一个简单树结构的java代码
时间: 2023-09-26 08:06:43 浏览: 53
### 回答1:
```java
public class Tree {
Node root;
public Tree() {
root = null;
}
public void addNode(int key, String name) {
Node newNode = new Node(key, name);
if (root == null) {
root = newNode;
} else {
Node focusNode = root;
Node parent;
while (true) {
parent = focusNode;
if (key < focusNode.key) {
focusNode = focusNode.leftChild;
if (focusNode == null) {
parent.leftChild = newNode;
return;
}
} else {
focusNode = focusNode.rightChild;
if (focusNode == null) {
parent.rightChild = newNode;
return;
}
}
}
}
}
public void inOrderTraverseTree(Node focusNode) {
if (focusNode != null) {
inOrderTraverseTree(focusNode.leftChild);
System.out.println(focusNode);
inOrderTraverseTree(focusNode.rightChild);
}
}
}class Node {
int key;
String name;
Node leftChild;
Node rightChild;
Node(int key, String name) {
this.key = key;
this.name = name;
}
public String toString() {
return name + " has a key " + key;
}
}
``答案:我可以为您生成一段简单树结构的Java代码,请看以下内容。
### 回答2:
以下是一个生成简单树结构的Java代码示例:
```java
import java.util.ArrayList;
import java.util.List;
class TreeNode {
private int val;
private List<TreeNode> children;
public TreeNode(int val) {
this.val = val;
this.children = new ArrayList<>();
}
public int getVal() {
return val;
}
public void addChild(TreeNode child) {
children.add(child);
}
public List<TreeNode> getChildren() {
return children;
}
}
public class TreeGenerator {
public static void main(String[] args) {
// 创建根节点
TreeNode root = new TreeNode(1);
// 创建子节点
TreeNode child1 = new TreeNode(2);
TreeNode child2 = new TreeNode(3);
TreeNode child3 = new TreeNode(4);
// 将子节点添加到根节点
root.addChild(child1);
root.addChild(child2);
root.addChild(child3);
// 创建孙子节点
TreeNode grandchild1 = new TreeNode(5);
TreeNode grandchild2 = new TreeNode(6);
// 将孙子节点添加到子节点
child1.addChild(grandchild1);
child1.addChild(grandchild2);
// 打印树结构
printTree(root, 0);
}
public static void printTree(TreeNode node, int level) {
// 打印节点值
for (int i = 0; i < level; i++) {
System.out.print(" ");
}
System.out.println(node.getVal());
// 递归打印子节点
for (TreeNode child : node.getChildren()) {
printTree(child, level + 1);
}
}
}
```
这段代码定义了一个`TreeNode`类,该类用于表示树的节点。每个节点包含一个整数值和一个子节点列表。`TreeGenerator`类包含一个`main`方法,在该方法中创建了一个简单的树结构,并调用`printTree`方法打印了整个树的结构。`printTree`方法使用递归方式遍历树的节点并打印每个节点的值。运行代码后,输出将是树的结构,按层级缩进显示。
### 回答3:
下面是一个生成简单树结构的Java代码示例:
```java
import java.util.ArrayList;
import java.util.List;
class TreeNode {
private int id;
private String name;
private List<TreeNode> children;
public TreeNode(int id, String name) {
this.id = id;
this.name = name;
this.children = new ArrayList<>();
}
public void addChild(TreeNode child) {
children.add(child);
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public List<TreeNode> getChildren() {
return children;
}
}
public class TreeExample {
public static void main(String[] args) {
TreeNode root = new TreeNode(1, "Root");
TreeNode child1 = new TreeNode(2, "Child 1");
TreeNode child2 = new TreeNode(3, "Child 2");
TreeNode child3 = new TreeNode(4, "Child 3");
TreeNode grandchild1 = new TreeNode(5, "Grandchild 1");
child1.addChild(grandchild1);
TreeNode grandchild2 = new TreeNode(6, "Grandchild 2");
child2.addChild(grandchild2);
root.addChild(child1);
root.addChild(child2);
root.addChild(child3);
System.out.println("Root: " + root.getName());
System.out.println("Children of Root:");
for (TreeNode child : root.getChildren()) {
System.out.println(child.getName());
for (TreeNode grandchild : child.getChildren()) {
System.out.println(" " + grandchild.getName());
}
}
}
}
```
这个例子中,我们定义了一个`TreeNode`类,它有一个唯一标识符和一个名称,以及一个子节点列表。我们还定义了一个`TreeExample`类,其中包含一个示例`main`方法来生成和遍历这个简单的树结构。
在`main`方法中,我们首先创建一个根节点对象。然后创建三个子节点对象,并将它们添加到根节点的子节点列表中。我们还为某些子节点创建了一些孙子节点,并将它们添加到相应的子节点的子节点列表中。
最后,在控制台上输出了这个树的结构,显示了根节点及其子节点和孙子节点的名称。
相关推荐


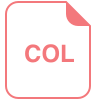
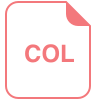










