用python写出一个手写识别数字项目,界面有画板,有清空和识别功能,按下识别按钮后能够把识别的数字结果显示出来
时间: 2024-03-06 17:48:45 浏览: 128
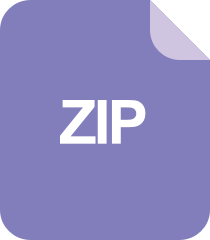
基于Python实现手写数字识别源码+文档说明+图形化界面.zip
好的,这个问题我可以回答。要实现这个项目,你需要掌握以下技能:
1. Python编程基础
2. Python GUI编程(如Tkinter)
3. 数字识别算法(如卷积神经网络)
下面是一个简单的实现步骤:
1. 创建一个GUI窗口,包括一个画板和两个按钮(清空和识别)。
2. 使用Tkinter库中的Canvas组件作为画板,用户可以在上面手写数字。
3. 当用户按下清空按钮时,清空画板。
4. 当用户按下识别按钮时,使用数字识别算法对画板中的数字进行识别,并将结果显示在GUI窗口中。
以下是一个简单的示例代码,仅供参考:
```python
from tkinter import *
import tensorflow as tf
import numpy as np
class HandwritingRecognition:
def __init__(self, master):
self.master = master
self.model = tf.keras.models.load_model('model.h5')
self.create_widgets()
def create_widgets(self):
self.canvas = Canvas(self.master, width=280, height=280, bg='white')
self.canvas.grid(row=0, column=0, columnspan=2, padx=5, pady=5)
self.recognize_button = Button(self.master, text='识别', command=self.recognize)
self.recognize_button.grid(row=1, column=0, padx=5, pady=5)
self.clear_button = Button(self.master, text='清空', command=self.clear)
self.clear_button.grid(row=1, column=1, padx=5, pady=5)
def recognize(self):
img = self.get_image()
img = img.reshape((1, 28, 28, 1))
prediction = self.model.predict(img)
number = np.argmax(prediction)
result_label = Label(self.master, text='识别结果:{}'.format(number))
result_label.grid(row=2, column=0, columnspan=2)
def clear(self):
self.canvas.delete('all')
def get_image(self):
ps = self.canvas.postscript(colormode='gray')
img = tf.image.decode_png(ps.split('\n')[4:-3], channels=1)
img = tf.image.resize(img, (28, 28))
img = tf.image.convert_image_dtype(img, tf.float32)
img = 1 - img
img = tf.reshape(img, (28, 28))
return img.numpy()
if __name__ == '__main__':
root = Tk()
root.title('手写数字识别')
app = HandwritingRecognition(root)
root.mainloop()
```
在这个示例代码中,我们使用了TensorFlow库中的Keras模块来加载预训练的数字识别模型。当用户按下“识别”按钮时,我们将获取画板中的图像数据,将其转换为28x28的灰度图像,并将其输入到模型中进行预测。最后,我们在GUI窗口中显示识别结果。
阅读全文
相关推荐
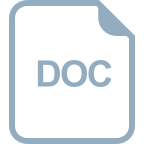
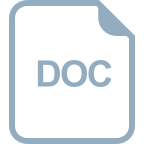
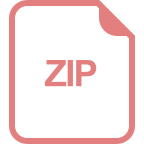
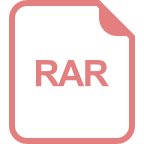
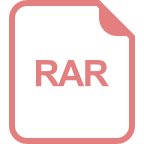
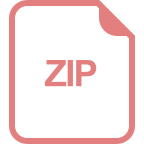
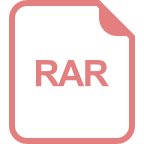
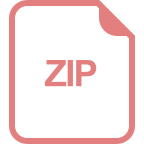
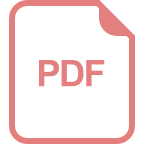
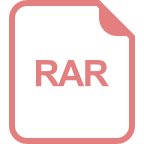
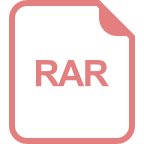
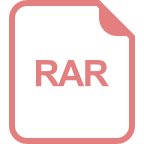
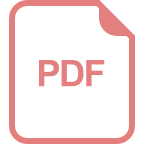
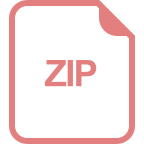
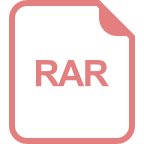
