C语言在学生信息单链表中查找指定姓名的学生成绩
时间: 2024-02-05 18:10:29 浏览: 57
假设你已经实现了一个学生信息单链表,每个节点包含学生姓名和成绩等信息。现在需要在该链表中查找指定姓名的学生成绩。
具体实现步骤如下:
1. 定义一个函数,接收两个参数:链表头指针和要查找的姓名。
2. 遍历链表,查找节点的姓名是否与要查找的姓名相同。
3. 如果相同,则返回该节点的成绩;否则继续遍历链表。
4. 如果遍历完整个链表仍未找到指定姓名的学生,则返回一个错误提示。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 学生信息结构体
typedef struct student {
char name[20]; // 姓名
int score; // 成绩
struct student* next; // 下一个节点指针
} Student;
// 在链表中查找指定姓名的学生成绩
int findScoreByName(Student* head, char* name) {
Student* p = head;
while (p != NULL) {
if (strcmp(p->name, name) == 0) { // 如果找到了指定姓名的学生
return p->score;
}
p = p->next;
}
// 如果遍历完整个链表仍未找到指定姓名的学生
printf("未找到姓名为%s的学生\n", name);
return -1; // 返回一个错误提示
}
int main() {
// 创建一个学生信息链表
Student* head = (Student*)malloc(sizeof(Student));
strcpy(head->name, "Alice");
head->score = 90;
Student* node1 = (Student*)malloc(sizeof(Student));
strcpy(node1->name, "Bob");
node1->score = 85;
head->next = node1;
Student* node2 = (Student*)malloc(sizeof(Student));
strcpy(node2->name, "Cindy");
node2->score = 92;
node1->next = node2;
node2->next = NULL;
// 查找指定姓名的学生成绩
int score = findScoreByName(head, "Bob");
printf("Bob的成绩为:%d\n", score);
// 释放链表内存
free(node2);
free(node1);
free(head);
return 0;
}
```
输出结果:
```
Bob的成绩为:85
```
阅读全文
相关推荐


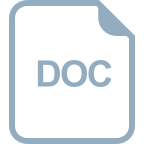
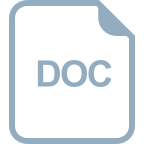
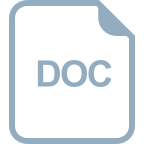
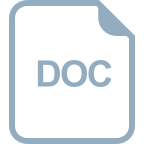
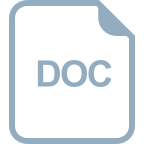
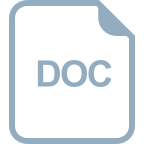
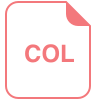





