定义学生结构体struct Student类型如下: char id[10]; //学号 char Name[20];//姓名 double Score[3];//3门课程成绩 在主函数定义3个struct Student类型变量,并赋值,将3个变量插入到单链表的表头、表尾和指定位置用,输入一个学号,在单链表上查找该学号的结点,找到,则删除该结点,找不到,则给出提示。C语言编写
时间: 2024-03-25 14:36:32 浏览: 11
以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
struct Student {
char id[10]; //学号
char Name[20];//姓名
double Score[3];//3门课程成绩
struct Student *next; //指向下一个节点的指针
};
// 创建新节点
struct Student *create_new_node(char id[], char Name[], double Score[]) {
struct Student *new_node = (struct Student*)malloc(sizeof(struct Student));
strcpy(new_node->id, id);
strcpy(new_node->Name, Name);
for (int i = 0; i < 3; i++) {
new_node->Score[i] = Score[i];
}
new_node->next = NULL;
return new_node;
}
// 将新节点插入到单链表的表头
struct Student *insert_to_head(struct Student *head, struct Student *new_node) {
new_node->next = head;
head = new_node;
return head;
}
// 将新节点插入到单链表的表尾
struct Student *insert_to_tail(struct Student *head, struct Student *new_node) {
if (head == NULL) {
head = new_node;
} else {
struct Student *p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = new_node;
}
return head;
}
// 将新节点插入到单链表的指定位置
struct Student *insert_to_pos(struct Student *head, struct Student *new_node, int pos) {
if (pos == 1) {
new_node->next = head;
head = new_node;
} else {
struct Student *p = head;
int i = 1;
while (p != NULL && i < pos - 1) {
p = p->next;
i++;
}
if (p == NULL) {
printf("插入位置无效\n");
} else {
new_node->next = p->next;
p->next = new_node;
}
}
return head;
}
// 在单链表上查找指定学号的结点,并删除该结点
struct Student *delete_by_id(struct Student *head, char id[]) {
struct Student *p = head;
struct Student *prev = NULL;
while (p != NULL && strcmp(p->id, id) != 0) {
prev = p;
p = p->next;
}
if (p == NULL) {
printf("未找到该学号的学生\n");
} else {
if (prev == NULL) { //要删除的节点是头结点
head = p->next;
} else {
prev->next = p->next;
}
free(p);
printf("删除成功\n");
}
return head;
}
int main() {
// 定义3个struct Student类型变量,并赋值
struct Student s1 = {"001", "张三", {85.5, 90, 88.5}, NULL};
struct Student s2 = {"002", "李四", {90, 92, 87}, NULL};
struct Student s3 = {"003", "王五", {91.5, 87, 89.5}, NULL};
// 将3个变量插入到单链表的表头、表尾和指定位置
struct Student *head = NULL;
head = insert_to_head(head, &s1);
head = insert_to_tail(head, &s2);
head = insert_to_pos(head, &s3, 2);
// 打印单链表所有节点
struct Student *p = head;
while (p != NULL) {
printf("学号:%s,姓名:%s,成绩:%lf %lf %lf\n", p->id, p->Name, p->Score[0], p->Score[1], p->Score[2]);
p = p->next;
}
// 输入一个学号,在单链表上查找该学号的结点,找到,则删除该结点,找不到,则给出提示。
char id[10];
printf("请输入要删除的学号:");
scanf("%s", id);
head = delete_by_id(head, id);
// 打印删除节点后的单链表所有节点
p = head;
while (p != NULL) {
printf("学号:%s,姓名:%s,成绩:%lf %lf %lf\n", p->id, p->Name, p->Score[0], p->Score[1], p->Score[2]);
p = p->next;
}
return 0;
}
```
相关推荐
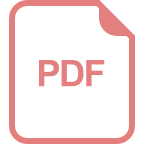
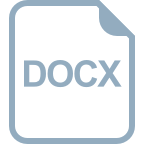
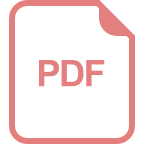




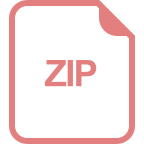
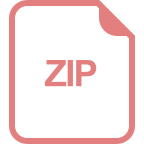
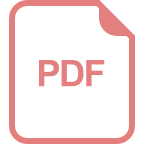
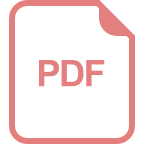
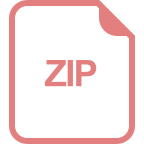
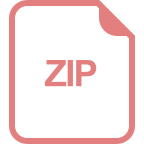
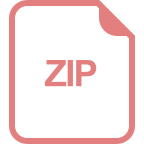
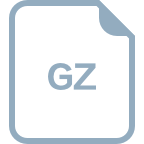
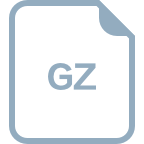
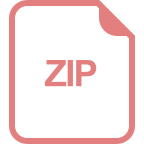