定义学生结构体struct Student类型如下: char id[10]; //学号 char Name[20];//姓名 double Score[3];//3门课程成绩 在主函数定义3个struct Student类型变量,并赋值,将3个变量插入到单链表的表头、表尾和指定位置
时间: 2024-01-21 18:01:53 浏览: 35
好的,我可以帮你实现这个功能。首先,我们需要定义一个结构体 `struct Student`,如下所示:
```c++
struct Student {
char id[10]; // 学号
char Name[20]; // 姓名
double Score[3]; // 3门课程成绩
};
```
接下来,我们需要定义一个单链表结构体 `struct ListNode`,如下所示:
```c++
struct ListNode {
Student data; // 存储数据
ListNode *next; // 指向下一个节点的指针
};
```
然后,我们可以定义一个函数 `insert`,用于将 `struct Student` 类型的变量插入到单链表中。该函数的参数包括:
- `ListNode *&head`:单链表头节点的引用
- `ListNode *&tail`:单链表尾节点的引用
- `int pos`:指定插入位置,如果 `pos` 为 `-1`,则插入到尾部
- `Student newStudent`:待插入的 `Student` 变量
函数的具体实现如下:
```c++
void insert(ListNode *&head, ListNode *&tail, int pos, Student newStudent) {
// 创建新节点
ListNode *newNode = new ListNode;
newNode->data = newStudent;
newNode->next = nullptr;
// 插入到头部
if (pos == 0) {
newNode->next = head;
head = newNode;
if (tail == nullptr) {
tail = head;
}
return;
}
// 插入到尾部
if (pos == -1) {
if (tail == nullptr) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
return;
}
// 插入到指定位置
ListNode *p = head;
int i = 0;
while (p != nullptr && i < pos - 1) {
p = p->next;
i++;
}
if (p == nullptr) {
cout << "插入位置无效" << endl;
return;
}
newNode->next = p->next;
p->next = newNode;
}
```
最后,在主函数中定义3个 `struct Student` 类型的变量,并分别插入到单链表的表头、表尾和指定位置,如下所示:
```c++
int main() {
ListNode *head = nullptr;
ListNode *tail = nullptr;
// 定义3个 Student 变量
Student stu1 = {"1001", "张三", {80, 85, 90}};
Student stu2 = {"1002", "李四", {75, 88, 92}};
Student stu3 = {"1003", "王五", {90, 92, 85}};
// 将 stu1 插入到表头
insert(head, tail, 0, stu1);
// 将 stu2 插入到表尾
insert(head, tail, -1, stu2);
// 将 stu3 插入到第二个位置
insert(head, tail, 1, stu3);
return 0;
}
```
这样,我们就可以将3个 `struct Student` 类型的变量分别插入到单链表的表头、表尾和指定位置了。
相关推荐
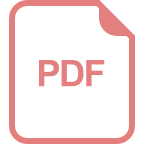
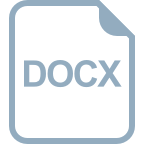
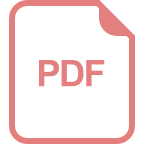



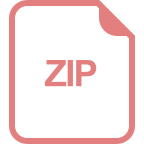
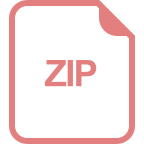
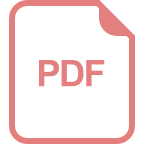
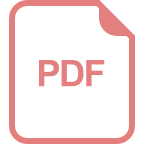
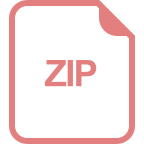
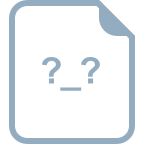
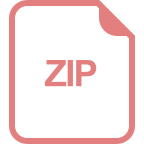
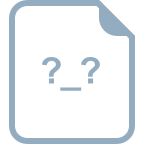
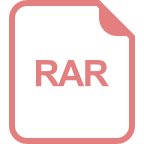