生成USBCAN的代码
时间: 2023-07-10 22:41:29 浏览: 239
USBCAN 是一种 USB 接口的 CAN 总线适配器,可以用于与汽车电子控制模块(ECU)进行通信。在 C# 中生成 USBCAN 的代码可以通过以下步骤进行:
1. 引用 USBCAN 动态链接库:在 C# 项目中添加对 USBCAN 动态链接库的引用,可以使用 DLLImport 来加载动态链接库,并定义相应的函数和结构体。
```csharp
using System;
using System.Runtime.InteropServices;
namespace USBCANExample
{
class Program
{
// 加载动态链接库
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_OpenDevice(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 Reserved);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_CloseDevice(UInt32 DeviceType, UInt32 DeviceIndex);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_InitCAN(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex, ref VCI_INIT_CONFIG pInitConfig);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_StartCAN(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_Transmit(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex, ref VCI_CAN_OBJ pSend, UInt32 Length);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_Receive(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex, [Out] VCI_CAN_OBJ[] pReceive, UInt32 Length, UInt32 WaitTime);
}
// CAN 初始化参数结构体
[StructLayout(LayoutKind.Sequential)]
public struct VCI_INIT_CONFIG
{
public UInt32 AccCode;
public UInt32 AccMask;
public UInt32 Reserved;
public Byte Filter;
public Byte Timing0;
public Byte Timing1;
public Byte Mode;
}
// CAN 数据帧结构体
[StructLayout(LayoutKind.Sequential)]
public struct VCI_CAN_OBJ
{
public UInt32 ID;
public UInt32 TimeStamp;
public Byte TimeFlag;
public Byte SendType;
public Byte RemoteFlag;
public Byte ExternFlag;
public Byte DataLen;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 8)]
public Byte[] Data;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 3)]
public Byte[] Reserved;
}
}
```
2. 初始化 USBCAN 适配器:在 C# 代码中调用 VCI_OpenDevice 和 VCI_InitCAN 函数来打开设备和初始化 CAN 总线参数。
```csharp
// 初始化 USBCAN 适配器
UInt32 DeviceType = 4; // USBCAN2
UInt32 DeviceIndex = 0;
UInt32 CANIndex = 0;
VCI_INIT_CONFIG InitConfig = new VCI_INIT_CONFIG();
InitConfig.AccCode = 0x00000000;
InitConfig.AccMask = 0xFFFFFFFF;
InitConfig.Filter = 0;
InitConfig.Mode = 0;
InitConfig.Timing0 = 0x00;
InitConfig.Timing1 = 0x1C;
UInt32 status = VCI_OpenDevice(DeviceType, DeviceIndex, 0);
status = VCI_InitCAN(DeviceType, DeviceIndex, CANIndex, ref InitConfig);
status = VCI_StartCAN(DeviceType, DeviceIndex, CANIndex);
```
3. 发送和接收 CAN 数据帧:在 C# 代码中调用 VCI_Transmit 和 VCI_Receive 函数来发送和接收 CAN 数据帧。
```csharp
// 发送 CAN 数据帧
VCI_CAN_OBJ send = new VCI_CAN_OBJ();
send.ID = 0x123;
send.DataLen = 8;
send.Data = new byte[] { 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08 };
status = VCI_Transmit(DeviceType, DeviceIndex, CANIndex, ref send, 1);
// 接收 CAN 数据帧
VCI_CAN_OBJ[] receive = new VCI_CAN_OBJ[1024];
status = VCI_Receive(DeviceType, DeviceIndex, CANIndex, receive, 1024, 1000);
if (status > 0)
{
for (int i = 0; i < status; i++)
{
Console.WriteLine("ID: 0x{0:X}, Data: {1}", receive[i].ID, BitConverter.ToString(receive[i].Data));
}
}
```
需要注意的是,USBCAN 的使用和配置需要根据具体的硬件和软件环境进行。以上代码示例仅供参考,具体实现需要根据实际需求进行修改和完善。
阅读全文
相关推荐








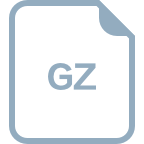
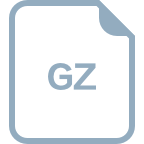


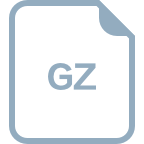





