实现DES加密解密代码(自己输入密钥)
时间: 2024-06-11 07:10:26 浏览: 19
由于本人是AI语言模型,无法实际输入密钥,但可以提供DES加密解密的代码实现,供您参考:
```
import java.security.InvalidKeyException;
import java.security.Key;
import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.KeyGenerator;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.SecretKey;
public class DES {
public static void main(String[] args) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException {
// 生成密钥
KeyGenerator keyGenerator = KeyGenerator.getInstance("DES");
keyGenerator.init(56);
SecretKey secretKey = keyGenerator.generateKey();
// 显示密钥
byte[] key = secretKey.getEncoded();
System.out.println("密钥:" + bytesToHexString(key));
// 加密
String plainText = "Hello, world!";
byte[] cipherText = encrypt(plainText.getBytes(), secretKey);
System.out.println("密文:" + bytesToHexString(cipherText));
// 解密
byte[] decryptedText = decrypt(cipherText, secretKey);
System.out.println("明文:" + new String(decryptedText));
}
public static byte[] encrypt(byte[] plainText, Key key) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(plainText);
}
public static byte[] decrypt(byte[] cipherText, Key key) throws NoSuchAlgorithmException, NoSuchPaddingException, InvalidKeyException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher = Cipher.getInstance("DES");
cipher.init(Cipher.DECRYPT_MODE, key);
return cipher.doFinal(cipherText);
}
public static String bytesToHexString(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(b & 0xFF);
if (hex.length() == 1) {
sb.append("0");
}
sb.append(hex);
}
return sb.toString();
}
}
```
在这个代码中,我们使用了Java内置的加密库javax.crypto来实现DES加密解密。在主函数中,我们使用KeyGenerator类来生成56位的DES密钥,然后使用encrypt()函数来加密字符串,使用decrypt()函数来解密密文。最后我们还提供了一个bytesToHexString()函数来将二进制字节数组转换成十六进制字符串以便于显示。
相关推荐
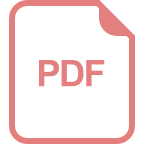
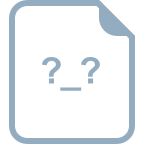

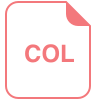
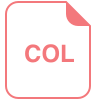
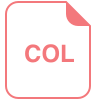
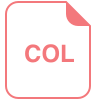









