找出字符串中出现次数最多的字符。例如:bbcaaa,出现次数最多的字符是a。 c
时间: 2024-05-01 16:17:16 浏览: 82
代码如下:
```python
def find_most_frequent_char(s):
char_dict = {}
for char in s:
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
max_count = 0
most_freq_char = ''
for char, count in char_dict.items():
if count > max_count:
max_count = count
most_freq_char = char
return most_freq_char
s = 'bbcaaa'
print(find_most_frequent_char(s)) # 输出a
```
相关问题
找出字符串中出现次数最多的字符。例如:bbcaaa,出现次数最多的字符是a。
可以用一个字典来记录每个字符出现的次数,然后遍历字典找出出现次数最多的字符。
代码如下:
```python
def find_most_common_char(s):
char_count = {}
for c in s:
char_count[c] = char_count.get(c, 0) + 1
max_count = 0
max_char = None
for c, count in char_count.items():
if count > max_count:
max_count = count
max_char = c
return max_char
```
测试代码:
```python
s = 'bbcaaa'
print(find_most_common_char(s)) # 输出'a'
```
c语言找出字符串中出现次数最多的字符
可以使用一个数组来记录每个字符出现的次数,然后遍历字符串,对应字符的计数器加一。最后再遍历一遍数组,找出计数器最大的字符即可。以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100];
int count[256] = {}; // 记录每个字符出现的次数,ASCII 码共有 256 个字符
printf("请输入一个字符串:");
scanf("%s", str);
int len = strlen(str);
for (int i = ; i < len; i++) {
count[str[i]]++; // 对应字符的计数器加一
}
int max_count = ;
char max_char;
for (int i = ; i < 256; i++) {
if (count[i] > max_count) {
max_count = count[i];
max_char = i;
}
}
printf("出现次数最多的字符是 %c,出现了 %d 次。\n", max_char, max_count);
return ;
}
```
阅读全文
相关推荐
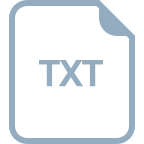
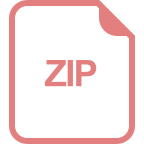
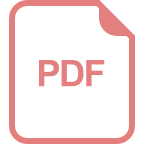
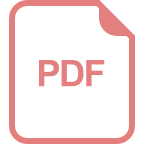









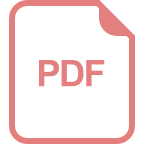