找出字符串中出现次数最多的字符,如果出现次数一样,输出靠前字符。python
时间: 2023-10-30 19:16:27 浏览: 95
可以使用字典来统计每个字符出现的次数,然后找出出现次数最多的字符即可。代码如下:
```python
def find_most_frequent_char(s):
# 统计每个字符出现的次数
char_count = {}
for c in s:
if c in char_count:
char_count[c] += 1
else:
char_count[c] = 1
# 找出出现次数最多的字符
max_count = 0
max_char = ''
for c, count in char_count.items():
if count > max_count:
max_count = count
max_char = c
return max_char
# 测试
s = 'abbcddd'
print(find_most_frequent_char(s)) # 输出 d
```
如果出现次数一样,那么我们可以用一个变量来记录当前出现次数最多的字符,然后遍历字典,如果有出现次数相同的字符,就比较它和当前最多的字符的位置,取靠前的字符。代码如下:
```python
def find_most_frequent_char(s):
# 统计每个字符出现的次数
char_count = {}
for c in s:
if c in char_count:
char_count[c] += 1
else:
char_count[c] = 1
# 找出出现次数最多的字符
max_count = 0
max_char = ''
for c, count in char_count.items():
if count > max_count:
max_count = count
max_char = c
elif count == max_count and s.index(c) < s.index(max_char):
max_char = c
return max_char
# 测试
s = 'abbcddd'
print(find_most_frequent_char(s)) # 输出 d
```
这样就能找出出现次数最多的字符了。
阅读全文
相关推荐
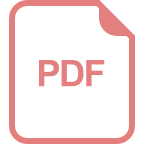
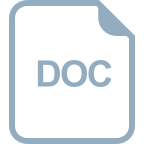












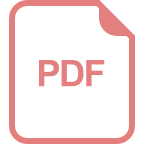