Elasticsearch继承springboot怎么创建索引
时间: 2024-01-07 19:03:43 浏览: 122
在Spring Boot中使用Elasticsearch,可以通过Elasticsearch的Java API来创建索引。下面是一个简单的示例,演示如何创建一个名为"books"的索引,包含title和author字段:
1. 添加Elasticsearch依赖
在pom.xml中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
```
2. 配置Elasticsearch连接
在application.properties文件中添加以下配置:
```properties
spring.data.elasticsearch.cluster-name=my-application
spring.data.elasticsearch.cluster-nodes=localhost:9300
```
3. 创建索引
创建索引的方法可以在任何Spring组件中使用,例如,一个Service或Controller。以下是一个简单的例子:
```java
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentType;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate;
import org.springframework.stereotype.Component;
@Component
public class BookIndexer {
@Autowired
private RestHighLevelClient client;
@Autowired
private ElasticsearchRestTemplate elasticsearchTemplate;
public void createIndex() throws IOException {
String indexName = "books";
String mapping = "{\n" +
" \"properties\": {\n" +
" \"title\": {\n" +
" \"type\": \"text\"\n" +
" },\n" +
" \"author\": {\n" +
" \"type\": \"text\"\n" +
" }\n" +
" }\n" +
"}";
boolean indexExists = client.indices().exists(new GetIndexRequest(indexName), RequestOptions.DEFAULT);
if (!indexExists) {
CreateIndexRequest createIndexRequest = new CreateIndexRequest(indexName);
createIndexRequest.mapping(mapping, XContentType.JSON);
CreateIndexResponse createIndexResponse = client.indices().create(createIndexRequest, RequestOptions.DEFAULT);
boolean acknowledged = createIndexResponse.isAcknowledged();
if (acknowledged) {
elasticsearchTemplate.refresh(indexName);
}
}
}
}
```
在这个例子中,我们注入了RestHighLevelClient和ElasticsearchRestTemplate,然后创建了一个名为"books"的索引,并定义了title和author字段。如果索引不存在,就创建一个新的索引。最后,我们刷新了索引以确保它被创建。
4. 测试
为了测试这个例子,可以编写一个简单的JUnit测试:
```java
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class BookIndexerTest {
@Autowired
private BookIndexer bookIndexer;
@Test
public void testCreateIndex() throws IOException {
bookIndexer.createIndex();
}
}
```
当运行测试时,如果一切正常,你应该会看到"books"索引被成功创建。
阅读全文
相关推荐
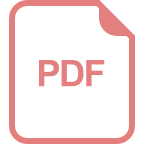
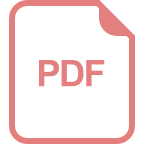
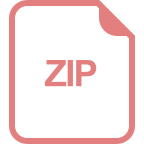

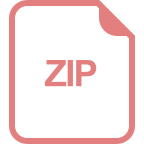
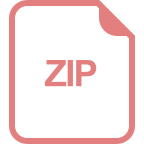



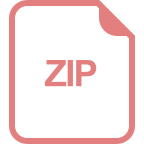
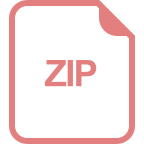
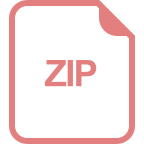
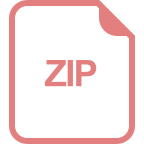
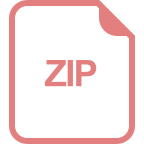
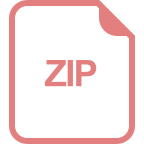
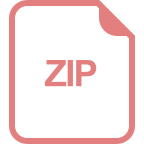
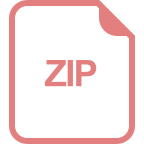
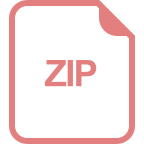
