BiLSTM_CRF(word2idx, tag2idx, Config.embedding_dim, Config.bilstm_hidden_dim).cuda()
时间: 2024-06-03 16:08:48 浏览: 129
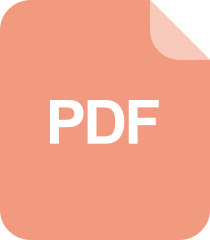
BiLSTM-CRF.
This code initializes a Bidirectional Long Short-Term Memory (BiLSTM) model with a Conditional Random Field (CRF) layer for named entity recognition (NER) or sequence labeling tasks.
The model takes as input a dictionary mapping words to their corresponding indices (word2idx) and a dictionary mapping tags to their corresponding indices (tag2idx). It also takes the embedding dimension and BiLSTM hidden dimension specified in the Config class.
The model is then moved to the GPU (cuda()) for faster training and inference.
阅读全文
相关推荐
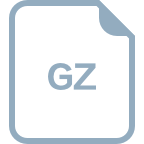
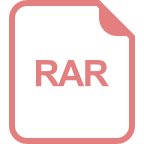

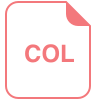





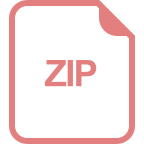
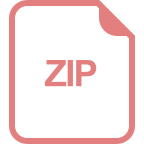
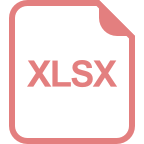
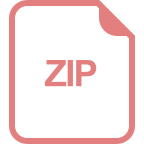
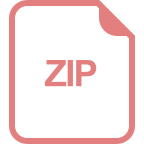