那么 我只需要对方法执行之前 用aop插入方法我最少需要用到那几个aop注解
时间: 2024-05-03 22:16:35 浏览: 9
如果你想在方法执行之前使用AOP插入方法,你需要使用`@Before`注解。除此之外,你可能还需要使用其他注解来定义切入点和切面,例如`@Pointcut`定义切入点,`@Aspect`定义切面。以下是一个示例代码:
```java
@Aspect
@Component
public class MyAspect {
@Before("execution(* com.example.MyService.*(..))")
public void beforeMethod(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().getName());
}
// other advice methods ...
}
```
在上面的示例中,`@Aspect`注解表示这是一个切面类,`@Before`注解表示这是一个前置通知,`execution(* com.example.MyService.*(..))`表示切入点,即`com.example.MyService`类中的所有方法。你需要将这个切面类声明为一个Spring组件,因此需要添加`@Component`注解。
相关问题
springaop 方法执行成功 改变注解的值
使用Spring AOP可以实现方法执行成功后改变注解的值。首先,需要在Spring配置文件中启用AOP的支持。然后,在目标方法所在的类上添加相关的注解,用于标识需要进行切面处理的方法。接下来,创建一个切面类,在切面类中定义一个环绕通知方法,该方法会在目标方法执行前后进行调用。在该环绕通知方法中,可以通过反射机制获取目标方法上的注解信息,并进行相应的处理。
假设有一个需要改变注解值的目标方法如下:
```java
@MyAnnotation("old value")
public void myMethod() {
// 目标方法的实现逻辑
}
```
首先,需要创建一个自定义的注解类`MyAnnotation`:
```java
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyAnnotation {
String value() default "";
}
```
然后,创建一个切面类`MyAspect`,并在该类中定义一个环绕通知方法`changeAnnotationValue`:
```java
@Component
@Aspect
public class MyAspect {
@Around("@annotation(myAnnotation)")
public Object changeAnnotationValue(ProceedingJoinPoint joinPoint, MyAnnotation myAnnotation) throws Throwable {
// 获取目标方法
MethodSignature methodSignature = (MethodSignature) joinPoint.getSignature();
Method targetMethod = methodSignature.getMethod();
// 获取目标方法上的注解
MyAnnotation annotation = targetMethod.getAnnotation(MyAnnotation.class);
// 修改注解值
MyAnnotation modifiedAnnotation = new MyAnnotationImpl(annotation.value() + " modified value");
// 反射机制修改注解值
MutableMethodMetadata mutableMetadata = new MutableMethodMetadata(targetMethod, false);
mutableMetadata.addAnnotation(modifiedAnnotation);
AnnotationUtils.synthesizeAndMergeAnnotations(modifiedAnnotation, mutableMetadata);
// 调用目标方法
Object result = joinPoint.proceed();
return result;
}
}
```
最后,在Spring配置文件中进行AOP的配置:
```xml
<aop:aspectj-autoproxy />
<context:annotation-config />
<context:component-scan base-package="com.example" />
```
这样,当目标方法`myMethod`被调用时,首先会执行切面类`MyAspect`中的环绕通知方法`changeAnnotationValue`,在该方法中修改了目标方法上`MyAnnotation`注解的值,然后再执行目标方法,实现了方法执行成功后改变注解的值。
java 异步方法中执行aop方法
在Java中,可以使用AOP框架来实现在异步方法中执行AOP方法。具体步骤如下:
1. 定义一个切面类,包含需要执行的AOP方法。
2. 在需要执行AOP方法的地方,使用AOP框架的@Aspect注解标识该类是一个切面类。
3. 使用AOP框架的@Around注解标识需要执行AOP方法的方法,在@Around注解中使用ProceedingJoinPoint参数来获取异步方法的执行信息。
4. 在@Around注解中,使用proceed()方法来执行异步方法,并在执行异步方法前后调用需要执行的AOP方法。
下面是一个简单的示例代码:
切面类:
```java
@Aspect
@Component
public class AsyncAspect {
@Around("@annotation(org.springframework.scheduling.annotation.Async)")
public Object doAround(ProceedingJoinPoint joinPoint) throws Throwable {
// 异步方法执行前调用的AOP方法
System.out.println("Before async method execution...");
// 执行异步方法
Object result = joinPoint.proceed();
// 异步方法执行后调用的AOP方法
System.out.println("After async method execution...");
return result;
}
}
```
异步方法:
```java
@Service
public class AsyncService {
@Async
public void asyncMethod() {
// 异步方法的具体实现
System.out.println("Async method is executed...");
}
}
```
在上面的示例中,@Aspect注解标识AsyncAspect类是一个切面类,@Around注解标识doAround()方法需要执行AOP方法的地方,使用ProceedingJoinPoint参数获取异步方法的执行信息,然后在执行异步方法前后调用需要执行的AOP方法。异步方法使用@Async注解标识,表示该方法是一个异步方法。
相关推荐
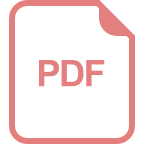
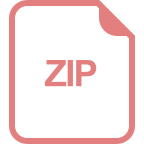
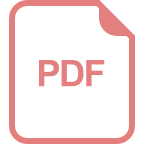
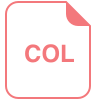
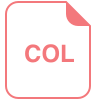
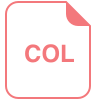
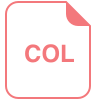
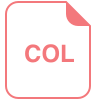







